SqlDataAdapter Event: updated and updating
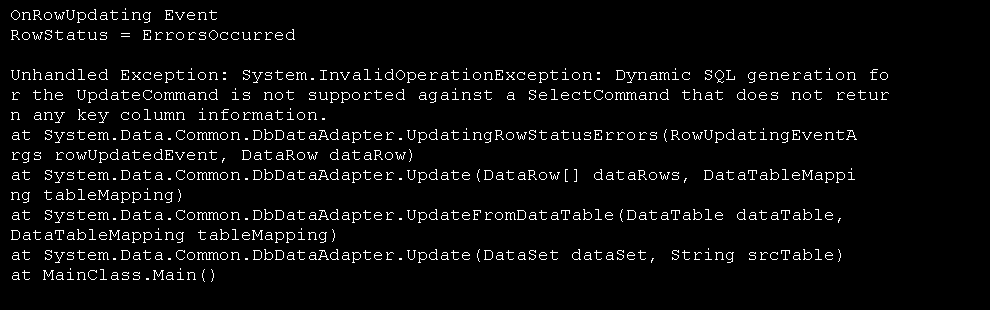
Imports System
Imports System.Data
Imports System.Data.SqlClient
public class MainClass
Shared Sub Main()
Dim thisConnection As New SqlConnection("server=(local)\SQLEXPRESS;" & _
"integrated security=sspi;database=MyDatabase")
Dim sql As String = "SELECT FirstName, LastName From Employee"
Try
thisConnection.Open()
Dim thisAdapter As New SqlDataAdapter(sql, thisConnection)
Dim cb As New SqlCommandBuilder(thisAdapter)
Dim ds As New DataSet
thisAdapter.Fill(ds, 0, 1, "Customers")
AddHandler thisAdapter.RowUpdating, AddressOf OnRowUpdating
AddHandler thisAdapter.RowUpdated, AddressOf OnRowUpdated
Dim dt As DataTable = ds.Tables("Customers")
dt.Rows(0)(1) = "The Volcano Corporation"
thisAdapter.Update(ds, "Customers")
RemoveHandler thisAdapter.RowUpdating, AddressOf OnRowUpdating
RemoveHandler thisAdapter.RowUpdated, AddressOf OnRowUpdated
Catch ex As SqlException
Console.WriteLine(ex.Message)
Finally
' Close Connection
thisConnection.Close()
End Try
End Sub
' Handler for OnRowUpdating
Shared Private Sub OnRowUpdating(ByVal sender As Object, ByVal e As SqlRowUpdatingEventArgs)
Console.WriteLine("OnRowUpdating Event")
If Not e.Status = UpdateStatus.Continue Then
Console.WriteLine("RowStatus = " & e.Status.ToString())
End If
End Sub
' Handler for OnRowUpdated
Shared Private Sub OnRowUpdated(ByVal sender As Object, ByVal e As SqlRowUpdatedEventArgs)
Console.WriteLine("OnRowUpdated Event")
Console.WriteLine("Records Affected = " & e.RecordsAffected.ToString())
End Sub
End Class
Related examples in the same category