Fill Reflection Data into ListView
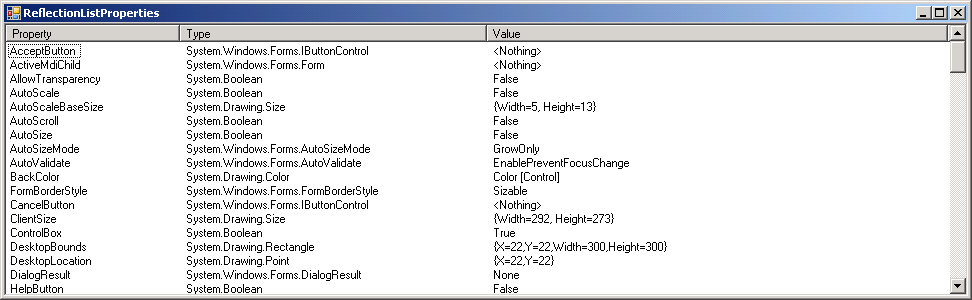
Imports System
Imports System.Collections
Imports System.Data
Imports System.Reflection
Imports System.Drawing
Imports System.Windows.Forms
Imports System.ComponentModel
Imports System.Drawing.Drawing2D
Imports System.IO
Public Class MainClass
Shared Sub Main()
Dim form1 As Form = New Form1
Application.Run(form1)
End Sub
End Class
Public Class Form1
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
' Make column headers.
lvwProperties.View = View.Details
lvwProperties.Columns.Clear()
lvwProperties.Columns.Add("Property", 10, HorizontalAlignment.Left)
lvwProperties.Columns.Add("Type", 10, HorizontalAlignment.Left)
lvwProperties.Columns.Add("Value", 10,HorizontalAlignment.Left)
Dim property_value As Object
Dim properties_info As PropertyInfo() = GetType(Form1).GetProperties()
lvwProperties.Items.Clear()
For i As Integer = 0 To properties_info.Length - 1
With properties_info(i)
If .GetIndexParameters().Length = 0 Then
property_value = .GetValue(Me, Nothing)
If property_value Is Nothing Then
ListViewMakeRow(lvwProperties, _
.Name, _
.PropertyType.ToString, _
"<Nothing>")
Else
ListViewMakeRow(lvwProperties, _
.Name, _
.PropertyType.ToString, _
property_value.ToString)
End If
Else
ListViewMakeRow(lvwProperties, _
.Name, _
.PropertyType.ToString, _
"<array>")
End If
End With
Next i
lvwProperties.Columns(0).Width = -2
lvwProperties.Columns(1).Width = -2
lvwProperties.Columns(2).Width = -2
Dim new_wid As Integer = 30
For i As Integer = 0 To lvwProperties.Columns.Count - 1
new_wid += lvwProperties.Columns(i).Width
Next i
Me.Size = New Size(new_wid, Me.Size.Height)
End Sub
Private Sub ListViewMakeRow(ByVal lvw As ListView, ByVal item_title As String, ByVal ParamArray subitem_titles() As String)
Dim new_item As ListViewItem = lvw.Items.Add(item_title)
For i As Integer = subitem_titles.GetLowerBound(0) To _
subitem_titles.GetUpperBound(0)
new_item.SubItems.Add(subitem_titles(i))
Next i
End Sub
End Class
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Partial Public Class Form1
Inherits System.Windows.Forms.Form
'Form overrides dispose to clean up the component list.
<System.Diagnostics.DebuggerNonUserCode()> _
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> _
Private Sub InitializeComponent()
Me.lvwProperties = New System.Windows.Forms.ListView
Me.SuspendLayout()
'
'lvwProperties
'
Me.lvwProperties.Dock = System.Windows.Forms.DockStyle.Fill
Me.lvwProperties.Location = New System.Drawing.Point(0, 0)
Me.lvwProperties.Name = "lvwProperties"
Me.lvwProperties.Size = New System.Drawing.Size(292, 273)
Me.lvwProperties.TabIndex = 1
'
'Form1
'
Me.AutoScaleDimensions = New System.Drawing.SizeF(6.0!, 13.0!)
Me.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font
Me.ClientSize = New System.Drawing.Size(292, 273)
Me.Controls.Add(Me.lvwProperties)
Me.Name = "Form1"
Me.Text = "ReflectionListProperties"
Me.ResumeLayout(False)
End Sub
Friend WithEvents lvwProperties As System.Windows.Forms.ListView
End Class
Related examples in the same category