Use Regular Expression to Validate Email address
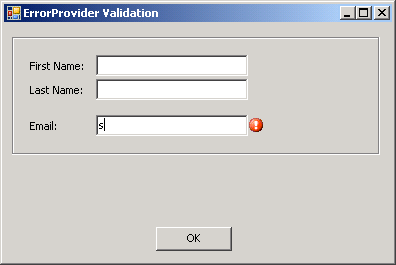
Imports System
Imports System.Drawing
Imports System.Windows.Forms
Public Class MainClass
Shared Sub Main()
Dim form1 As Form = New Form1
Application.Run(form1)
End Sub
End Class
Public Class Form1
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
Friend WithEvents GroupBox1 As System.Windows.Forms.GroupBox
Friend WithEvents Label2 As System.Windows.Forms.Label
Friend WithEvents Label1 As System.Windows.Forms.Label
Friend WithEvents txtFirstName As System.Windows.Forms.TextBox
Friend WithEvents txtLastName As System.Windows.Forms.TextBox
Friend WithEvents Button1 As System.Windows.Forms.Button
Friend WithEvents errProvider As System.Windows.Forms.ErrorProvider
Friend WithEvents txtEmail As System.Windows.Forms.TextBox
Friend WithEvents Label3 As System.Windows.Forms.Label
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.GroupBox1 = New System.Windows.Forms.GroupBox()
Me.Label2 = New System.Windows.Forms.Label()
Me.Label1 = New System.Windows.Forms.Label()
Me.txtFirstName = New System.Windows.Forms.TextBox()
Me.txtLastName = New System.Windows.Forms.TextBox()
Me.Button1 = New System.Windows.Forms.Button()
Me.errProvider = New System.Windows.Forms.ErrorProvider()
Me.txtEmail = New System.Windows.Forms.TextBox()
Me.Label3 = New System.Windows.Forms.Label()
Me.GroupBox1.SuspendLayout()
Me.SuspendLayout()
'
'GroupBox1
'
Me.GroupBox1.Controls.AddRange(New System.Windows.Forms.Control() {Me.Label3, Me.txtEmail, Me.Label2, Me.Label1, Me.txtFirstName, Me.txtLastName})
Me.GroupBox1.Location = New System.Drawing.Point(8, 8)
Me.GroupBox1.Name = "GroupBox1"
Me.GroupBox1.Size = New System.Drawing.Size(368, 124)
Me.GroupBox1.TabIndex = 11
Me.GroupBox1.TabStop = False
'
'Label2
'
Me.Label2.Location = New System.Drawing.Point(16, 52)
Me.Label2.Name = "Label2"
Me.Label2.Size = New System.Drawing.Size(64, 16)
Me.Label2.TabIndex = 8
Me.Label2.Text = "Last Name:"
'
'Label1
'
Me.Label1.Location = New System.Drawing.Point(16, 28)
Me.Label1.Name = "Label1"
Me.Label1.Size = New System.Drawing.Size(64, 16)
Me.Label1.TabIndex = 7
Me.Label1.Text = "First Name:"
'
'txtFirstName
'
Me.txtFirstName.Location = New System.Drawing.Point(84, 24)
Me.txtFirstName.Name = "txtFirstName"
Me.txtFirstName.Size = New System.Drawing.Size(152, 21)
Me.txtFirstName.TabIndex = 4
Me.txtFirstName.Text = ""
'
'txtLastName
'
Me.txtLastName.Location = New System.Drawing.Point(84, 48)
Me.txtLastName.Name = "txtLastName"
Me.txtLastName.Size = New System.Drawing.Size(152, 21)
Me.txtLastName.TabIndex = 5
Me.txtLastName.Text = ""
'
'Button1
'
Me.Button1.Location = New System.Drawing.Point(152, 204)
Me.Button1.Name = "Button1"
Me.Button1.Size = New System.Drawing.Size(76, 24)
Me.Button1.TabIndex = 10
Me.Button1.Text = "OK"
'
'txtEmail
'
Me.txtEmail.Location = New System.Drawing.Point(84, 84)
Me.txtEmail.Name = "txtEmail"
Me.txtEmail.Size = New System.Drawing.Size(152, 21)
Me.txtEmail.TabIndex = 9
Me.txtEmail.Text = ""
'
'Label3
'
Me.Label3.Location = New System.Drawing.Point(16, 88)
Me.Label3.Name = "Label3"
Me.Label3.Size = New System.Drawing.Size(64, 16)
Me.Label3.TabIndex = 10
Me.Label3.Text = "Email:"
'
'ErrorProviderValidation
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 14)
Me.ClientSize = New System.Drawing.Size(388, 238)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.GroupBox1, Me.Button1})
Me.Font = New System.Drawing.Font("Tahoma", 8.25!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.Name = "ErrorProviderValidation"
Me.Text = "ErrorProvider Validation"
Me.GroupBox1.ResumeLayout(False)
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub txtEmail_KeyPress(ByVal sender As Object, ByVal e As System.Windows.Forms.KeyPressEventArgs) Handles txtEmail.KeyPress
Dim Expression As New System.Text.RegularExpressions.Regex("\S+@\S+\.\S+")
If Expression.IsMatch(CType(sender, TextBox).Text) Then
errProvider.SetError(sender, "")
Else
errProvider.SetError(sender, "Not a valid email.")
End If
End Sub
End Class
Related examples in the same category