Click Button to Set Status Bar Text
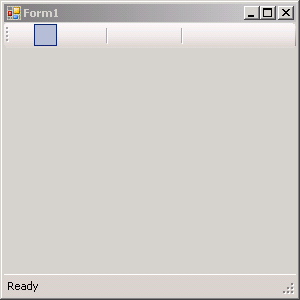
Imports System
Imports System.Collections
Imports System.ComponentModel
Imports System.Windows.Forms
Imports System.Data
Imports System.Configuration
Imports System.Resources
Imports System.Drawing
Imports System.Drawing.Drawing2D
Public Class MainClass
Shared Sub Main()
Dim myform As Form = New Form1()
Application.Run(myform)
End Sub
End Class
Public Class Form1
Private Sub copyToolStripButton_Click(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles copyToolStripButton.Click
'Update the status bar
sspStatus.Text = "The Copy button was clicked."
End Sub
Private Sub cutToolStripButton_Click(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles cutToolStripButton.Click
'Update the status bar
sspStatus.Text = "The Cut button was clicked."
End Sub
Private Sub helpToolStripButton_Click(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles helpToolStripButton.Click
'Update the status bar
sspStatus.Text = "The Help button was clicked."
End Sub
Private Sub newToolStripButton_Click(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles newToolStripButton.Click
'Update the status bar
sspStatus.Text = "The New button was clicked."
End Sub
Private Sub openToolStripButton_Click(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles openToolStripButton.Click
'Update the status bar
sspStatus.Text = "The Open button was clicked."
End Sub
Private Sub pasteToolStripButton_Click(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles pasteToolStripButton.Click
'Update the status bar
sspStatus.Text = "The Paste button was clicked."
End Sub
Private Sub printToolStripButton_Click(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles printToolStripButton.Click
'Update the status bar
sspStatus.Text = "The Print button was clicked."
End Sub
Private Sub saveToolStripButton_Click(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles saveToolStripButton.Click
'Update the status bar
sspStatus.Text = "The Save button was clicked."
End Sub
End Class
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Partial Public Class Form1
Inherits System.Windows.Forms.Form
'Form overrides dispose to clean up the component list.
<System.Diagnostics.DebuggerNonUserCode()> _
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> _
Private Sub InitializeComponent()
' Dim resources As System.ComponentModel.ComponentResourceManager = New System.ComponentModel.ComponentResourceManager(GetType(Form1))
Me.ToolStrip1 = New System.Windows.Forms.ToolStrip
Me.newToolStripButton = New System.Windows.Forms.ToolStripButton
Me.openToolStripButton = New System.Windows.Forms.ToolStripButton
Me.saveToolStripButton = New System.Windows.Forms.ToolStripButton
Me.printToolStripButton = New System.Windows.Forms.ToolStripButton
Me.toolStripSeparator = New System.Windows.Forms.ToolStripSeparator
Me.cutToolStripButton = New System.Windows.Forms.ToolStripButton
Me.copyToolStripButton = New System.Windows.Forms.ToolStripButton
Me.pasteToolStripButton = New System.Windows.Forms.ToolStripButton
Me.toolStripSeparator1 = New System.Windows.Forms.ToolStripSeparator
Me.helpToolStripButton = New System.Windows.Forms.ToolStripButton
Me.StatusStrip1 = New System.Windows.Forms.StatusStrip
Me.sspStatus = New System.Windows.Forms.ToolStripStatusLabel
Me.ToolStrip1.SuspendLayout()
Me.StatusStrip1.SuspendLayout()
Me.SuspendLayout()
'
'ToolStrip1
'
Me.ToolStrip1.Items.AddRange(New System.Windows.Forms.ToolStripItem() {Me.newToolStripButton, Me.openToolStripButton, Me.saveToolStripButton, Me.printToolStripButton, Me.toolStripSeparator, Me.cutToolStripButton, Me.copyToolStripButton, Me.pasteToolStripButton, Me.toolStripSeparator1, Me.helpToolStripButton})
Me.ToolStrip1.Location = New System.Drawing.Point(0, 0)
Me.ToolStrip1.Name = "ToolStrip1"
Me.ToolStrip1.Size = New System.Drawing.Size(292, 25)
Me.ToolStrip1.TabIndex = 0
Me.ToolStrip1.Text = "ToolStrip1"
'
'newToolStripButton
'
Me.newToolStripButton.DisplayStyle = System.Windows.Forms.ToolStripItemDisplayStyle.Image
' Me.newToolStripButton.Image = CType(resources.GetObject("newToolStripButton.Image"), System.Drawing.Image)
Me.newToolStripButton.ImageTransparentColor = System.Drawing.Color.Magenta
Me.newToolStripButton.Name = "newToolStripButton"
Me.newToolStripButton.Text = "&New"
'
'openToolStripButton
'
Me.openToolStripButton.DisplayStyle = System.Windows.Forms.ToolStripItemDisplayStyle.Image
' Me.openToolStripButton.Image = CType(resources.GetObject("openToolStripButton.Image"), System.Drawing.Image)
Me.openToolStripButton.ImageTransparentColor = System.Drawing.Color.Magenta
Me.openToolStripButton.Name = "openToolStripButton"
Me.openToolStripButton.Text = "&Open"
'
'saveToolStripButton
'
Me.saveToolStripButton.DisplayStyle = System.Windows.Forms.ToolStripItemDisplayStyle.Image
' Me.saveToolStripButton.Image = CType(resources.GetObject("saveToolStripButton.Image"), System.Drawing.Image)
Me.saveToolStripButton.ImageTransparentColor = System.Drawing.Color.Magenta
Me.saveToolStripButton.Name = "saveToolStripButton"
Me.saveToolStripButton.Text = "&Save"
'
'printToolStripButton
'
Me.printToolStripButton.DisplayStyle = System.Windows.Forms.ToolStripItemDisplayStyle.Image
' Me.printToolStripButton.Image = CType(resources.GetObject("printToolStripButton.Image"), System.Drawing.Image)
Me.printToolStripButton.ImageTransparentColor = System.Drawing.Color.Magenta
Me.printToolStripButton.Name = "printToolStripButton"
Me.printToolStripButton.Text = "&Print"
'
'toolStripSeparator
'
Me.toolStripSeparator.Name = "toolStripSeparator"
'
'cutToolStripButton
'
Me.cutToolStripButton.DisplayStyle = System.Windows.Forms.ToolStripItemDisplayStyle.Image
' Me.cutToolStripButton.Image = CType(resources.GetObject("cutToolStripButton.Image"), System.Drawing.Image)
Me.cutToolStripButton.ImageTransparentColor = System.Drawing.Color.Magenta
Me.cutToolStripButton.Name = "cutToolStripButton"
Me.cutToolStripButton.Text = "C&ut"
'
'copyToolStripButton
'
Me.copyToolStripButton.DisplayStyle = System.Windows.Forms.ToolStripItemDisplayStyle.Image
' Me.copyToolStripButton.Image = CType(resources.GetObject("copyToolStripButton.Image"), System.Drawing.Image)
Me.copyToolStripButton.ImageTransparentColor = System.Drawing.Color.Magenta
Me.copyToolStripButton.Name = "copyToolStripButton"
Me.copyToolStripButton.Text = "&Copy"
'
'pasteToolStripButton
'
Me.pasteToolStripButton.DisplayStyle = System.Windows.Forms.ToolStripItemDisplayStyle.Image
' Me.pasteToolStripButton.Image = CType(resources.GetObject("pasteToolStripButton.Image"), System.Drawing.Image)
Me.pasteToolStripButton.ImageTransparentColor = System.Drawing.Color.Magenta
Me.pasteToolStripButton.Name = "pasteToolStripButton"
Me.pasteToolStripButton.Text = "&Paste"
'
'toolStripSeparator1
'
Me.toolStripSeparator1.Name = "toolStripSeparator1"
'
'helpToolStripButton
'
Me.helpToolStripButton.DisplayStyle = System.Windows.Forms.ToolStripItemDisplayStyle.Image
' Me.helpToolStripButton.Image = CType(resources.GetObject("helpToolStripButton.Image"), System.Drawing.Image)
Me.helpToolStripButton.ImageTransparentColor = System.Drawing.Color.Magenta
Me.helpToolStripButton.Name = "helpToolStripButton"
Me.helpToolStripButton.Text = "He&lp"
'
'StatusStrip1
'
Me.StatusStrip1.Items.AddRange(New System.Windows.Forms.ToolStripItem() {Me.sspStatus})
Me.StatusStrip1.LayoutStyle = System.Windows.Forms.ToolStripLayoutStyle.Table
Me.StatusStrip1.Location = New System.Drawing.Point(0, 251)
Me.StatusStrip1.Name = "StatusStrip1"
Me.StatusStrip1.Size = New System.Drawing.Size(292, 22)
Me.StatusStrip1.TabIndex = 1
Me.StatusStrip1.Text = "StatusStrip1"
'
'sspStatus
'
Me.sspStatus.DisplayStyle = System.Windows.Forms.ToolStripItemDisplayStyle.Text
Me.sspStatus.Name = "sspStatus"
Me.sspStatus.Text = "Ready"
'
'Form1
'
Me.AutoScaleDimensions = New System.Drawing.SizeF(6.0!, 13.0!)
Me.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font
Me.ClientSize = New System.Drawing.Size(292, 273)
Me.Controls.Add(Me.ToolStrip1)
Me.Controls.Add(Me.StatusStrip1)
Me.Name = "Form1"
Me.Text = "Form1"
Me.ToolStrip1.ResumeLayout(False)
Me.StatusStrip1.ResumeLayout(False)
Me.ResumeLayout(False)
Me.PerformLayout()
End Sub
Friend WithEvents ToolStrip1 As System.Windows.Forms.ToolStrip
Friend WithEvents newToolStripButton As System.Windows.Forms.ToolStripButton
Friend WithEvents openToolStripButton As System.Windows.Forms.ToolStripButton
Friend WithEvents saveToolStripButton As System.Windows.Forms.ToolStripButton
Friend WithEvents printToolStripButton As System.Windows.Forms.ToolStripButton
Friend WithEvents toolStripSeparator As System.Windows.Forms.ToolStripSeparator
Friend WithEvents cutToolStripButton As System.Windows.Forms.ToolStripButton
Friend WithEvents copyToolStripButton As System.Windows.Forms.ToolStripButton
Friend WithEvents pasteToolStripButton As System.Windows.Forms.ToolStripButton
Friend WithEvents toolStripSeparator1 As System.Windows.Forms.ToolStripSeparator
Friend WithEvents helpToolStripButton As System.Windows.Forms.ToolStripButton
Friend WithEvents StatusStrip1 As System.Windows.Forms.StatusStrip
Friend WithEvents sspStatus As System.Windows.Forms.ToolStripStatusLabel
End Class
Related examples in the same category