Custom Tab control
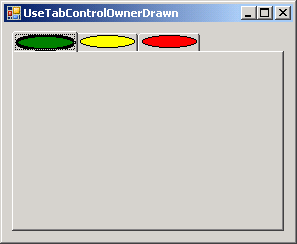
Imports System
Imports System.Data
Imports System.Windows.Forms
Imports System.Drawing
Imports System.Data.SqlClient
public class MainClass
Shared Sub Main()
Dim form1 As Form = New Form1
Application.Run(form1)
End Sub
End Class
Public Class Form1
Private Sub tabProject_DrawItem(ByVal sender As Object, _
ByVal e As System.Windows.Forms.DrawItemEventArgs) Handles tabProject.DrawItem
Dim rect As Rectangle = e.Bounds
If (e.State And DrawItemState.Selected) = DrawItemState.Selected Then
rect.X += 4
rect.Y += 4
rect.Width -= 8
rect.Height -= 8
Else
rect.X += 2
rect.Y += 2
rect.Width -= 4
rect.Height -= 4
End If
Select Case e.Index
Case 0
e.Graphics.FillEllipse(Brushes.Green, rect)
Case 1
e.Graphics.FillEllipse(Brushes.Yellow, rect)
Case 2
e.Graphics.FillEllipse(Brushes.Red, rect)
End Select
If (e.State And DrawItemState.Selected) = DrawItemState.Selected Then
e.Graphics.DrawEllipse( _
New Pen(Color.Black, 3), _
rect)
Else
e.Graphics.DrawEllipse(Pens.Black, rect)
End If
End Sub
End Class
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Partial Public Class Form1
Inherits System.Windows.Forms.Form
'Form overrides dispose to clean up the component list.
<System.Diagnostics.DebuggerNonUserCode()> _
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> _
Private Sub InitializeComponent()
Me.tabProject = New System.Windows.Forms.TabControl
Me.TabPage1 = New System.Windows.Forms.TabPage
Me.TabPage2 = New System.Windows.Forms.TabPage
Me.TabPage3 = New System.Windows.Forms.TabPage
Me.tabProject.SuspendLayout()
Me.SuspendLayout()
'
'tabProject
'
Me.tabProject.Controls.Add(Me.TabPage1)
Me.tabProject.Controls.Add(Me.TabPage2)
Me.tabProject.Controls.Add(Me.TabPage3)
Me.tabProject.DrawMode = System.Windows.Forms.TabDrawMode.OwnerDrawFixed
Me.tabProject.Location = New System.Drawing.Point(8, 8)
Me.tabProject.Name = "tabProject"
Me.tabProject.SelectedIndex = 0
Me.tabProject.Size = New System.Drawing.Size(272, 200)
Me.tabProject.TabIndex = 1
'
'TabPage1
'
Me.TabPage1.Location = New System.Drawing.Point(4, 22)
Me.TabPage1.Name = "TabPage1"
Me.TabPage1.Padding = New System.Windows.Forms.Padding(3)
Me.TabPage1.Size = New System.Drawing.Size(264, 174)
Me.TabPage1.TabIndex = 0
Me.TabPage1.Text = "TabPage1"
'
'TabPage2
'
Me.TabPage2.Location = New System.Drawing.Point(4, 22)
Me.TabPage2.Name = "TabPage2"
Me.TabPage2.Padding = New System.Windows.Forms.Padding(3)
Me.TabPage2.Size = New System.Drawing.Size(264, 174)
Me.TabPage2.TabIndex = 1
Me.TabPage2.Text = "TabPage2"
'
'TabPage3
'
Me.TabPage3.Location = New System.Drawing.Point(4, 22)
Me.TabPage3.Name = "TabPage3"
Me.TabPage3.Size = New System.Drawing.Size(264, 174)
Me.TabPage3.TabIndex = 2
Me.TabPage3.Text = "TabPage3"
'
'Form1
'
Me.AutoScaleDimensions = New System.Drawing.SizeF(6.0!, 13.0!)
Me.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font
Me.ClientSize = New System.Drawing.Size(289, 217)
Me.Controls.Add(Me.tabProject)
Me.Name = "Form1"
Me.Text = "UseTabControlOwnerDrawn"
Me.tabProject.ResumeLayout(False)
Me.ResumeLayout(False)
End Sub
Friend WithEvents tabProject As System.Windows.Forms.TabControl
Friend WithEvents TabPage1 As System.Windows.Forms.TabPage
Friend WithEvents TabPage2 As System.Windows.Forms.TabPage
Friend WithEvents TabPage3 As System.Windows.Forms.TabPage
End Class
Related examples in the same category