Displaying directories and their contents in ListView
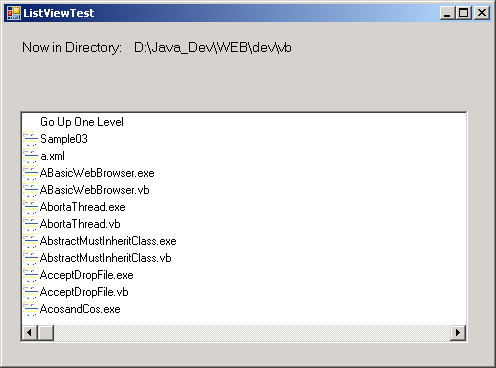
Imports System
Imports System.Drawing
Imports System.Windows.Forms
Imports System.IO
Public Class MainClass
Shared Sub Main()
Dim myform As Form = New FrmListView()
Application.Run(myform)
End Sub ' Main
End Class
Public Class FrmListView
Inherits Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
' display labels for current location in directory tree
Friend WithEvents lblCurrent As Label
Friend WithEvents lblDisplay As Label
' displays contents of current directory
Friend WithEvents lvwBrowser As ListView
' specifies images for file icons and folder icons
Friend WithEvents ilsFileFolder As ImageList
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.components = New System.ComponentModel.Container()
Me.ilsFileFolder = New System.Windows.Forms.ImageList(Me.components)
Me.lvwBrowser = New System.Windows.Forms.ListView()
Me.lblCurrent = New System.Windows.Forms.Label()
Me.lblDisplay = New System.Windows.Forms.Label()
Me.SuspendLayout()
'
'ilsFileFolder
'
Me.ilsFileFolder.ColorDepth = System.Windows.Forms.ColorDepth.Depth8Bit
Me.ilsFileFolder.ImageSize = New System.Drawing.Size(16, 16)
Me.ilsFileFolder.TransparentColor = System.Drawing.Color.Transparent
'
'lvwBrowser
'
Me.lvwBrowser.Location = New System.Drawing.Point(16, 88)
Me.lvwBrowser.Name = "lvwBrowser"
Me.lvwBrowser.RightToLeft = System.Windows.Forms.RightToLeft.No
Me.lvwBrowser.Size = New System.Drawing.Size(448, 232)
Me.lvwBrowser.SmallImageList = Me.ilsFileFolder
Me.lvwBrowser.TabIndex = 2
Me.lvwBrowser.View = System.Windows.Forms.View.List
'
'lblCurrent
'
Me.lblCurrent.Font = New System.Drawing.Font("Microsoft Sans Serif", 9.75!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.lblCurrent.ForeColor = System.Drawing.SystemColors.WindowText
Me.lblCurrent.Location = New System.Drawing.Point(16, 16)
Me.lblCurrent.Name = "lblCurrent"
Me.lblCurrent.Size = New System.Drawing.Size(112, 23)
Me.lblCurrent.TabIndex = 0
Me.lblCurrent.Text = "Now in Directory:"
'
'lblDisplay
'
Me.lblDisplay.Font = New System.Drawing.Font("Microsoft Sans Serif", 9.75!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.lblDisplay.ForeColor = System.Drawing.SystemColors.WindowText
Me.lblDisplay.Location = New System.Drawing.Point(128, 16)
Me.lblDisplay.Name = "lblDisplay"
Me.lblDisplay.Size = New System.Drawing.Size(344, 56)
Me.lblDisplay.TabIndex = 1
'
'FrmListView
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(488, 341)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.lvwBrowser, Me.lblDisplay, Me.lblCurrent})
Me.Name = "FrmListView"
Me.Text = "ListViewTest"
Me.ResumeLayout(False)
End Sub
#End Region
Dim currentDirectory As String = Directory.GetCurrentDirectory()
Private Sub lvwBrowser_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles lvwBrowser.Click
If lvwBrowser.SelectedItems.Count <> 0 Then
If lvwBrowser.Items(0).Selected Then
Dim directoryObject As DirectoryInfo = _
New DirectoryInfo(currentDirectory)
If Not (directoryObject.Parent Is Nothing) Then
LoadFilesInDirectory(directoryObject.Parent.FullName)
End If
Else
Dim chosen As String = lvwBrowser.SelectedItems(0).Text
If Directory.Exists(currentDirectory & "\" & chosen) Then
If currentDirectory = "C:\" Then
LoadFilesInDirectory(currentDirectory & chosen)
Else
LoadFilesInDirectory(currentDirectory & _
"\" & chosen)
End If
End If
End If
lblDisplay.Text = currentDirectory
End If
End Sub
Public Sub LoadFilesInDirectory _
(ByVal currentDirectoryValue As String)
Try
lvwBrowser.Items.Clear()
lvwBrowser.Items.Add("Go Up One Level")
currentDirectory = currentDirectoryValue
Dim newCurrentDirectory As DirectoryInfo = _
New DirectoryInfo(currentDirectory)
Dim directoryArray As DirectoryInfo() = _
newCurrentDirectory.GetDirectories()
Dim fileArray As FileInfo() = _
newCurrentDirectory.GetFiles()
Dim dir As DirectoryInfo
For Each dir In directoryArray
Dim newDirectoryItem As ListViewItem = _
lvwBrowser.Items.Add(dir.Name)
newDirectoryItem.ImageIndex = 0
Next
Dim file As FileInfo
For Each file In fileArray
Dim newFileItem As ListViewItem = _
lvwBrowser.Items.Add(file.Name)
newFileItem.ImageIndex = 1 ' set file image
Next
Catch exception As UnauthorizedAccessException
MessageBox.Show("Warning: Some files may " & _
"not be visible due to " + "permission settings", _
"Attention", 0, MessageBoxIcon.Warning)
End Try
End Sub ' LoadFilesInDirectory
' handle load event when Form displayed for first time
Private Sub FrmListView_Load(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles MyBase.Load
' set image list
Dim folderImage As Image = Image.FromFile("figure2.bmp")
Dim fileImage As Image = Image.FromFile("figure2.bmp")
ilsFileFolder.Images.Add(folderImage)
ilsFileFolder.Images.Add(fileImage)
' load current directory into browserListView
LoadFilesInDirectory(currentDirectory)
lblDisplay.Text = currentDirectory
End Sub ' FrmListView_Load
End Class
Related examples in the same category