Docking in VB
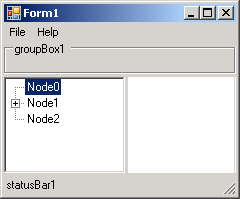
Imports System
Imports System.Drawing
Imports System.Collections
Imports System.ComponentModel
Imports System.Windows.Forms
Imports System.Data
Imports System.Configuration
Imports System.Resources
Public Class Form1
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
Friend WithEvents mainMenu1 As System.Windows.Forms.MainMenu
Friend WithEvents menuItem1 As System.Windows.Forms.MenuItem
Friend WithEvents menuItem2 As System.Windows.Forms.MenuItem
Friend WithEvents menuItem3 As System.Windows.Forms.MenuItem
Friend WithEvents menuItem4 As System.Windows.Forms.MenuItem
Friend WithEvents listView1 As System.Windows.Forms.ListView
Friend WithEvents splitter2 As System.Windows.Forms.Splitter
Friend WithEvents treeView1 As System.Windows.Forms.TreeView
Friend WithEvents splitter1 As System.Windows.Forms.Splitter
Friend WithEvents statusBar1 As System.Windows.Forms.StatusBar
Friend WithEvents groupBox1 As System.Windows.Forms.GroupBox
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Dim ListViewItem1 As System.Windows.Forms.ListViewItem = New System.Windows.Forms.ListViewItem(New System.Windows.Forms.ListViewItem.ListViewSubItem() {New System.Windows.Forms.ListViewItem.ListViewSubItem(Nothing, "Item 0", System.Drawing.SystemColors.WindowText, System.Drawing.SystemColors.Window, New System.Drawing.Font("Microsoft Sans Serif", 8.25!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte)))}, -1)
Dim ListViewItem2 As System.Windows.Forms.ListViewItem = New System.Windows.Forms.ListViewItem(New System.Windows.Forms.ListViewItem.ListViewSubItem() {New System.Windows.Forms.ListViewItem.ListViewSubItem(Nothing, "Item 1", System.Drawing.SystemColors.WindowText, System.Drawing.SystemColors.Window, New System.Drawing.Font("Microsoft Sans Serif", 8.25!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte)))}, -1)
Dim ListViewItem3 As System.Windows.Forms.ListViewItem = New System.Windows.Forms.ListViewItem(New System.Windows.Forms.ListViewItem.ListViewSubItem() {New System.Windows.Forms.ListViewItem.ListViewSubItem(Nothing, "Item 2", System.Drawing.SystemColors.WindowText, System.Drawing.SystemColors.Window, New System.Drawing.Font("Microsoft Sans Serif", 8.25!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte)))}, -1)
Dim ListViewItem4 As System.Windows.Forms.ListViewItem = New System.Windows.Forms.ListViewItem(New System.Windows.Forms.ListViewItem.ListViewSubItem() {New System.Windows.Forms.ListViewItem.ListViewSubItem(Nothing, "Item 3", System.Drawing.SystemColors.WindowText, System.Drawing.SystemColors.Window, New System.Drawing.Font("Microsoft Sans Serif", 8.25!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte)))}, -1)
Me.mainMenu1 = New System.Windows.Forms.MainMenu()
Me.menuItem1 = New System.Windows.Forms.MenuItem()
Me.menuItem2 = New System.Windows.Forms.MenuItem()
Me.menuItem3 = New System.Windows.Forms.MenuItem()
Me.menuItem4 = New System.Windows.Forms.MenuItem()
Me.listView1 = New System.Windows.Forms.ListView()
Me.splitter2 = New System.Windows.Forms.Splitter()
Me.treeView1 = New System.Windows.Forms.TreeView()
Me.splitter1 = New System.Windows.Forms.Splitter()
Me.statusBar1 = New System.Windows.Forms.StatusBar()
Me.groupBox1 = New System.Windows.Forms.GroupBox()
Me.SuspendLayout()
'
'mainMenu1
'
Me.mainMenu1.MenuItems.AddRange(New System.Windows.Forms.MenuItem() {Me.menuItem1, Me.menuItem3})
'
'menuItem1
'
Me.menuItem1.Index = 0
Me.menuItem1.MenuItems.AddRange(New System.Windows.Forms.MenuItem() {Me.menuItem2})
Me.menuItem1.Text = "&File"
'
'menuItem2
'
Me.menuItem2.Index = 0
Me.menuItem2.Text = "E&xit"
'
'menuItem3
'
Me.menuItem3.Index = 1
Me.menuItem3.MenuItems.AddRange(New System.Windows.Forms.MenuItem() {Me.menuItem4})
Me.menuItem3.Text = "&Help"
'
'menuItem4
'
Me.menuItem4.Index = 0
Me.menuItem4.Text = "&About..."
'
'listView1
'
Me.listView1.Dock = System.Windows.Forms.DockStyle.Fill
Me.listView1.Items.AddRange(New System.Windows.Forms.ListViewItem() {ListViewItem1, ListViewItem2, ListViewItem3, ListViewItem4})
Me.listView1.Name = "listView1"
Me.listView1.Size = New System.Drawing.Size(232, 153)
Me.listView1.TabIndex = 9
Me.listView1.View = System.Windows.Forms.View.List
'
'splitter2
'
Me.splitter2.Location = New System.Drawing.Point(121, 35)
Me.splitter2.Name = "splitter2"
Me.splitter2.Size = New System.Drawing.Size(3, 96)
Me.splitter2.TabIndex = 11
Me.splitter2.TabStop = False
'
'treeView1
'
Me.treeView1.Dock = System.Windows.Forms.DockStyle.Left
Me.treeView1.ImageIndex = -1
Me.treeView1.Location = New System.Drawing.Point(0, 35)
Me.treeView1.Name = "treeView1"
Me.treeView1.Nodes.AddRange(New System.Windows.Forms.TreeNode() {New System.Windows.Forms.TreeNode("Node0"), New System.Windows.Forms.TreeNode("Node1", New System.Windows.Forms.TreeNode() {New System.Windows.Forms.TreeNode("Node3", New System.Windows.Forms.TreeNode() {New System.Windows.Forms.TreeNode("Node4", New System.Windows.Forms.TreeNode() {New System.Windows.Forms.TreeNode("Node5")})})}), New System.Windows.Forms.TreeNode("Node2")})
Me.treeView1.SelectedImageIndex = -1
Me.treeView1.Size = New System.Drawing.Size(121, 96)
Me.treeView1.TabIndex = 7
'
'splitter1
'
Me.splitter1.Dock = System.Windows.Forms.DockStyle.Top
Me.splitter1.Location = New System.Drawing.Point(0, 32)
Me.splitter1.Name = "splitter1"
Me.splitter1.Size = New System.Drawing.Size(232, 3)
Me.splitter1.TabIndex = 8
Me.splitter1.TabStop = False
'
'statusBar1
'
Me.statusBar1.Location = New System.Drawing.Point(0, 131)
Me.statusBar1.Name = "statusBar1"
Me.statusBar1.Size = New System.Drawing.Size(232, 22)
Me.statusBar1.TabIndex = 6
Me.statusBar1.Text = "statusBar1"
'
'groupBox1
'
Me.groupBox1.Dock = System.Windows.Forms.DockStyle.Top
Me.groupBox1.Name = "groupBox1"
Me.groupBox1.Size = New System.Drawing.Size(232, 32)
Me.groupBox1.TabIndex = 10
Me.groupBox1.TabStop = False
Me.groupBox1.Text = "groupBox1"
'
'Form1
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(232, 153)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.splitter2, Me.treeView1, Me.splitter1, Me.statusBar1, Me.groupBox1, Me.listView1})
Me.Menu = Me.mainMenu1
Me.Name = "Form1"
Me.Text = "Form1"
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub menuItem2_Click(ByVal sender As Object, ByVal e As System.EventArgs) Handles menuItem2.Click
Me.Close()
End Sub
Private Sub menuItem4_Click(ByVal sender As Object, ByVal e As System.EventArgs) Handles menuItem4.Click
MessageBox.Show("Info", "About...")
End Sub
Shared Sub Main()
Dim myform As Form = New Form1()
Application.Run(myform)
End Sub
End Class
Related examples in the same category