List Box and Spinner Demo
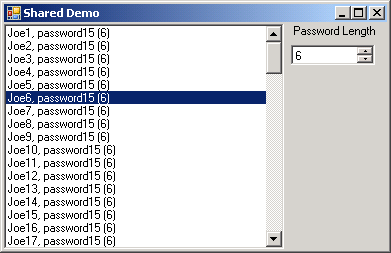
Imports System
Imports System.Collections
Imports System.ComponentModel
Imports System.Windows.Forms
Imports System.Data
Imports System.Configuration
Imports System.Resources
Imports System.Drawing
Imports System.Drawing.Drawing2D
Imports System.IO
Imports System.Drawing.Printing
Public Class MainClass
Shared Sub Main()
Dim myform As Form = New Form1()
Application.Run(myform)
End Sub
End Class
Public Class User
'Public members
Public Username As String
Public Shared MinPasswordLength As Integer = 6
'Private members
Private _password As String
'Password property
Public Property Password() As String
Get
Return _password
End Get
Set(ByVal value As String)
If value.Length >= MinPasswordLength Then
_password = value
End If
End Set
End Property
Public Shared Function CreateUser(ByVal userName As String, _
ByVal password As String) As User
'Delcare a new User object
Dim objUser As New User()
'Set the User properties
objUser.Username = userName
objUser.Password = password
'Return the new user
Return objUser
End Function
End Class
Public Class Form1
'Private member
Private arrUserList As New ArrayList()
Private Sub UpdateDisplay()
'Clear the list
lstUsers.Items.Clear()
'Add the users to the list box
For Each objUser As User In arrUserList
lstUsers.Items.Add(objUser.Username & ", " & objUser.Password & _
" (" & User.MinPasswordLength & ")")
Next
End Sub
Private Sub Form1_Load(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles Me.Load
'Load 100 users
For intIndex As Integer = 1 To 100
'Create a new user
Dim objUser As New User
objUser = User.CreateUser("Joe" & intIndex, "password15")
'Add the user to the array list
arrUserList.Add(objUser)
Next
'Update the display
UpdateDisplay()
End Sub
Private Sub nupMinPasswordLength_ValueChanged(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles nupMinPasswordLength.ValueChanged
'Set the minimum password length
User.MinPasswordLength = nupMinPasswordLength.Value
'Update the display
UpdateDisplay()
End Sub
End Class
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Partial Public Class Form1
Inherits System.Windows.Forms.Form
'Form overrides dispose to clean up the component list.
<System.Diagnostics.DebuggerNonUserCode()> _
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> _
Private Sub InitializeComponent()
Me.nupMinPasswordLength = New System.Windows.Forms.NumericUpDown
Me.Label1 = New System.Windows.Forms.Label
Me.lstUsers = New System.Windows.Forms.ListBox
CType(Me.nupMinPasswordLength, System.ComponentModel.ISupportInitialize).BeginInit()
Me.SuspendLayout()
'
'nupMinPasswordLength
'
Me.nupMinPasswordLength.Location = New System.Drawing.Point(287, 22)
Me.nupMinPasswordLength.Maximum = New Decimal(New Integer() {10, 0, 0, 0})
Me.nupMinPasswordLength.Name = "nupMinPasswordLength"
Me.nupMinPasswordLength.Size = New System.Drawing.Size(84, 20)
Me.nupMinPasswordLength.TabIndex = 5
Me.nupMinPasswordLength.Value = New Decimal(New Integer() {6, 0, 0, 0})
'
'Label1
'
Me.Label1.AutoSize = True
Me.Label1.Location = New System.Drawing.Point(287, 1)
Me.Label1.Name = "Label1"
Me.Label1.Size = New System.Drawing.Size(85, 13)
Me.Label1.TabIndex = 4
Me.Label1.Text = "Password Length"
'
'lstUsers
'
Me.lstUsers.FormattingEnabled = True
Me.lstUsers.IntegralHeight = False
Me.lstUsers.Location = New System.Drawing.Point(0, 1)
Me.lstUsers.Name = "lstUsers"
Me.lstUsers.Size = New System.Drawing.Size(280, 225)
Me.lstUsers.TabIndex = 3
'
'Form1
'
Me.AutoScaleDimensions = New System.Drawing.SizeF(6.0!, 13.0!)
Me.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font
Me.ClientSize = New System.Drawing.Size(383, 226)
Me.Controls.Add(Me.nupMinPasswordLength)
Me.Controls.Add(Me.Label1)
Me.Controls.Add(Me.lstUsers)
Me.Name = "Form1"
Me.Text = "Shared Demo"
CType(Me.nupMinPasswordLength, System.ComponentModel.ISupportInitialize).EndInit()
Me.ResumeLayout(False)
Me.PerformLayout()
End Sub
Friend WithEvents nupMinPasswordLength As System.Windows.Forms.NumericUpDown
Friend WithEvents Label1 As System.Windows.Forms.Label
Friend WithEvents lstUsers As System.Windows.Forms.ListBox
End Class
Related examples in the same category