Sub class UserControl to create your own control
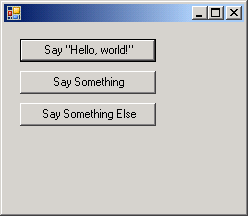
Imports System
Imports System.Collections
Imports System.Data
Imports System.IO
Imports System.Xml.Serialization
Imports System.Xml
Imports System.Windows.Forms
Imports System.Data.SqlClient
Imports System.Data.OleDb
Public Class MainClass
Shared Sub Main()
Dim form1 As Form = New Form1
Application.Run(form1)
End Sub
End Class
Public Class Form1
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
Friend WithEvents MyControl1 As MyControl
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.MyControl1 = New MyControl()
Me.SuspendLayout()
'
'MyControl1
'
Me.MyControl1.Location = New System.Drawing.Point(8, 8)
Me.MyControl1.MessageText = "Hello, world!"
Me.MyControl1.Name = "MyControl1"
Me.MyControl1.Size = New System.Drawing.Size(152, 104)
Me.MyControl1.TabIndex = 0
'
'Form1
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(240, 189)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.MyControl1})
Me.Name = "Form1"
Me.ResumeLayout(False)
End Sub
#End Region
End Class
Public Class MyControl
Inherits System.Windows.Forms.UserControl
Private _messageText As String
Event HelloWorld(ByVal sender As Object, ByVal e As System.EventArgs)
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
MessageText = "Hello, world!"
End Sub
'UserControl overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
Friend WithEvents btnSayHello As System.Windows.Forms.Button
Friend WithEvents btnSaySomething As System.Windows.Forms.Button
Friend WithEvents btnSaySomethingElse As System.Windows.Forms.Button
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.btnSayHello = New System.Windows.Forms.Button()
Me.btnSaySomething = New System.Windows.Forms.Button()
Me.btnSaySomethingElse = New System.Windows.Forms.Button()
Me.SuspendLayout()
'
'btnSayHello
'
Me.btnSayHello.Location = New System.Drawing.Point(8, 8)
Me.btnSayHello.Name = "btnSayHello"
Me.btnSayHello.Size = New System.Drawing.Size(136, 23)
Me.btnSayHello.TabIndex = 0
Me.btnSayHello.Text = "Say, ""Hello, world!"""
'
'btnSaySomething
'
Me.btnSaySomething.Location = New System.Drawing.Point(8, 40)
Me.btnSaySomething.Name = "btnSaySomething"
Me.btnSaySomething.Size = New System.Drawing.Size(136, 23)
Me.btnSaySomething.TabIndex = 1
Me.btnSaySomething.Text = "Say Something"
'
'btnSaySomethingElse
'
Me.btnSaySomethingElse.Location = New System.Drawing.Point(8, 72)
Me.btnSaySomethingElse.Name = "btnSaySomethingElse"
Me.btnSaySomethingElse.Size = New System.Drawing.Size(136, 23)
Me.btnSaySomethingElse.TabIndex = 2
Me.btnSaySomethingElse.Text = "Say Something Else"
'
'MyControl
'
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.btnSaySomethingElse, Me.btnSaySomething, Me.btnSayHello})
Me.Name = "MyControl"
Me.Size = New System.Drawing.Size(152, 104)
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub btnSayHello_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnSayHello.Click
' raise the event...
RaiseEvent HelloWorld(Me, New System.EventArgs())
MsgBox(_messageText)
End Sub
Private Sub btnSaySomething_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnSaySomething.Click
MsgBox("Something!")
End Sub
Private Sub btnSaySomethingElse_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnSaySomethingElse.Click
MsgBox("Something else!")
End Sub
Public Property MessageText() As String
Get
Return _messageText
End Get
Set(ByVal Value As String)
' set the text...
_messageText = Value
' update the button...
btnSayHello.Text = "Say """ & _messageText & """"
End Set
End Property
Public Sub ResetMessageText()
MessageText = "Hello, world!"
End Sub
Private Sub MyControl_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
End Sub
End Class
Related examples in the same category