The use of MDI parent and child windows
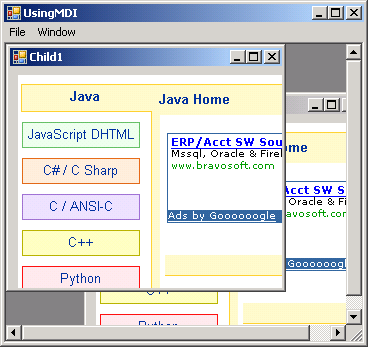
Imports System
Imports System.Drawing
Imports System.Windows.Forms
Imports System.IO
Public Class MainClass
Shared Sub Main()
Dim myform As Form = New FrmUsingMDI()
Application.Run(myform)
End Sub ' Main
End Class
Public Class FrmUsingMDI
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
' main menu containing menu items File and Window
Friend WithEvents mnuMain As MainMenu
' menu containing submenu New and menu item Exit
Friend WithEvents mnuitmFile As MenuItem
Friend WithEvents mnuitmExit As MenuItem
' submenu New
Friend WithEvents mnuitmNew As MenuItem
Friend WithEvents mnuitmChild1 As MenuItem
Friend WithEvents mnuitmChild2 As MenuItem
Friend WithEvents mnuitmChild3 As MenuItem
' menu containing menu items Cascade, TileHorizontal and
' TileVertical
Friend WithEvents mnuitmWindow As MenuItem
Friend WithEvents mnuitmCascade As MenuItem
Friend WithEvents mnuitmTileHorizontal As MenuItem
Friend WithEvents mnuitmTileVertical As MenuItem
'Required by the Windows Form Designer
Private components As System.ComponentModel.Container
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.mnuitmTileHorizontal = New System.Windows.Forms.MenuItem()
Me.mnuitmTileVertical = New System.Windows.Forms.MenuItem()
Me.mnuitmChild1 = New System.Windows.Forms.MenuItem()
Me.mnuitmCascade = New System.Windows.Forms.MenuItem()
Me.mnuitmChild2 = New System.Windows.Forms.MenuItem()
Me.mnuMain = New System.Windows.Forms.MainMenu()
Me.mnuitmFile = New System.Windows.Forms.MenuItem()
Me.mnuitmNew = New System.Windows.Forms.MenuItem()
Me.mnuitmChild3 = New System.Windows.Forms.MenuItem()
Me.mnuitmExit = New System.Windows.Forms.MenuItem()
Me.mnuitmWindow = New System.Windows.Forms.MenuItem()
'
'mnuitmTileHorizontal
'
Me.mnuitmTileHorizontal.Index = 1
Me.mnuitmTileHorizontal.Text = "Tile Horizontal"
'
'mnuitmTileVertical
'
Me.mnuitmTileVertical.Index = 2
Me.mnuitmTileVertical.Text = "Tile Vertical"
'
'mnuitmChild1
'
Me.mnuitmChild1.Index = 0
Me.mnuitmChild1.Text = "Child1"
'
'mnuitmCascade
'
Me.mnuitmCascade.Index = 0
Me.mnuitmCascade.Text = "Cascade"
'
'mnuitmChild2
'
Me.mnuitmChild2.Index = 1
Me.mnuitmChild2.Text = "Child2"
'
'mnuMain
'
Me.mnuMain.MenuItems.AddRange(New System.Windows.Forms.MenuItem() {Me.mnuitmFile, Me.mnuitmWindow})
'
'mnuitmFile
'
Me.mnuitmFile.Index = 0
Me.mnuitmFile.MenuItems.AddRange(New System.Windows.Forms.MenuItem() {Me.mnuitmNew, Me.mnuitmExit})
Me.mnuitmFile.Text = "File"
'
'mnuitmNew
'
Me.mnuitmNew.Index = 0
Me.mnuitmNew.MenuItems.AddRange(New System.Windows.Forms.MenuItem() {Me.mnuitmChild1, Me.mnuitmChild2, Me.mnuitmChild3})
Me.mnuitmNew.Text = "New"
'
'mnuitmChild3
'
Me.mnuitmChild3.Index = 2
Me.mnuitmChild3.Text = "Child3"
'
'mnuitmExit
'
Me.mnuitmExit.Index = 1
Me.mnuitmExit.Text = "Exit"
'
'mnuitmWindow
'
Me.mnuitmWindow.Index = 1
Me.mnuitmWindow.MdiList = True
Me.mnuitmWindow.MenuItems.AddRange(New System.Windows.Forms.MenuItem() {Me.mnuitmCascade, Me.mnuitmTileHorizontal, Me.mnuitmTileVertical})
Me.mnuitmWindow.Text = "Window"
'
'FrmUsingMDI
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(360, 301)
Me.IsMdiContainer = True
Me.Menu = Me.mnuMain
Me.Name = "FrmUsingMDI"
Me.Text = "UsingMDI"
End Sub
#End Region
Private childWindow As FrmChild
' create FrmChild1 when menu clicked
Private Sub mnuitmChild1_Click( _
ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles mnuitmChild1.Click
' create image path
Dim imagePath As String = "figure2.bmp"
' create new child
childWindow = New FrmChild(imagePath, "Child1")
childWindow.MdiParent = Me ' set parent
childWindow.Show() ' display child
End Sub ' mnuitmChild1_Click
' create FrmChild2 when menu clicked
Private Sub mnuitmChild2_Click( _
ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles mnuitmChild2.Click
' create image path
Dim imagePath As String = "figure2.bmp"
' create new child
childWindow = New FrmChild(imagePath, "Child2")
childWindow.MdiParent = Me ' set parent
childWindow.Show() ' display child
End Sub ' mnuitmChild2_Click
' create FrmChild3 when menu clicked
Private Sub mnuitmChild3_Click( _
ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles mnuitmChild3.Click
' create image path
Dim imagePath As String = "figure2.bmp"
' create new child
childWindow = New FrmChild(imagePath, "Child3")
childWindow.MdiParent = Me ' set parent
childWindow.Show() ' display child
End Sub ' mnuitmChild3_Click
Private Sub mnuitmExit_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles mnuitmExit.Click
Application.Exit()
End Sub ' mnuitmExit_Click
Private Sub mnuitmCascade_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles mnuitmCascade.Click
Me.LayoutMdi(MdiLayout.Cascade)
End Sub ' mnuitmCascade_Click
Private Sub mnuitmTileHorizontal_Click _
(ByVal sender As System.Object, ByVal e As System.EventArgs) _
Handles mnuitmTileHorizontal.Click
Me.LayoutMdi(MdiLayout.TileHorizontal)
End Sub ' mnuitmTileHorizontal_Click
Private Sub mnuitmTileVertical_Click _
(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles mnuitmTileVertical.Click
Me.LayoutMdi(MdiLayout.TileVertical)
End Sub
End Class
Public Class FrmChild
Inherits Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
' Public Sub New(ByVal imageInformation As String, ByVal windowName As String)
' Display(imageInformation, windowName)
' End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
' contains an image loaded from disk
Friend WithEvents picDisplay As PictureBox
'Required by the Windows Form Designer
Private components As System.ComponentModel.Container
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.picDisplay = New System.Windows.Forms.PictureBox()
Me.SuspendLayout()
'
'picDisplay
'
Me.picDisplay.Location = New System.Drawing.Point(8, 8)
Me.picDisplay.Name = "picDisplay"
Me.picDisplay.Size = New System.Drawing.Size(256, 208)
Me.picDisplay.SizeMode = System.Windows.Forms.PictureBoxSizeMode.AutoSize
Me.picDisplay.TabIndex = 0
Me.picDisplay.TabStop = False
'
'FrmChild1
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(272, 221)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.picDisplay})
Me.Name = "FrmChild"
Me.Text = ""
Me.ResumeLayout(False)
End Sub
#End Region
' constructor
Public Sub New(ByVal picture As String, ByVal name As String)
' call Visual Studio generated default constructor
Me.New()
' set title
Me.Text = name
' set image for picture box
picDisplay.Image = Image.FromFile(picture)
End Sub ' New
End Class
Related examples in the same category