Use Property Grid
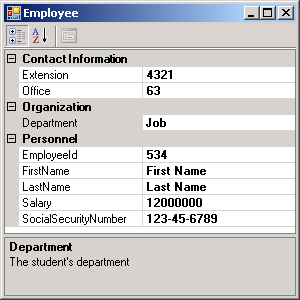
Imports System
Imports System.ComponentModel
Imports System.Windows.Forms
Public Class MainClass
Shared Sub Main(ByVal args As String())
Dim myform As Form = New Form1()
Application.Run(myform)
End Sub
End Class
Public Class Form1
Private m_Employee As Employee
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
m_Employee = New Employee("First Name", "Last Name", _
534, "Job", 63, 4321, _
"123-45-6789", 12000000)
PropertyGrid1.CommandsVisibleIfAvailable = True
PropertyGrid1.Text = "Student"
PropertyGrid1.SelectedObject = m_Employee
End Sub
End Class
Public Class Employee
Private m_FirstName As String
Private m_LastName As String
Private m_EmployeeId As Integer
Private m_Department As String
Private m_Office As Integer
Private m_Extension As Integer
Private m_SocialSecurityNumber As String
Private m_Salary As Integer
<Description("The student's extension"), _
Category("Contact Information")> _
Public Property Extension() As Integer
Get
Return m_Extension
End Get
Set(ByVal value As Integer)
m_Extension = value
End Set
End Property
<Description("The student's office number"), _
Category("Contact Information")> _
Public Property Office() As Integer
Get
Return m_Office
End Get
Set(ByVal value As Integer)
m_Office = value
End Set
End Property
<Description("The student's department"), _
Category("Organization")> _
Public Property Department() As String
Get
Return m_Department
End Get
Set(ByVal value As String)
m_Department = value
End Set
End Property
<Description("The student's ID"), _
Category("Personnel")> _
Public Property EmployeeId() As Integer
Get
Return m_EmployeeId
End Get
Set(ByVal value As Integer)
m_EmployeeId = value
End Set
End Property
<Description("The student's first name"), _
Category("Personnel")> _
Public Property FirstName() As String
Get
Return m_FirstName
End Get
Set(ByVal value As String)
m_FirstName = value
End Set
End Property
<Description("The student's last name"), _
Category("Personnel")> _
Public Property LastName() As String
Get
Return m_LastName
End Get
Set(ByVal value As String)
m_LastName = value
End Set
End Property
<Description("The student's Social Security number"), _
Category("Personnel")> _
Public Property SocialSecurityNumber() As String
Get
Return m_SocialSecurityNumber
End Get
Set(ByVal value As String)
m_SocialSecurityNumber = value
End Set
End Property
<Description("The student's annual salary"), _
Category("Personnel")> _
Public Property Salary() As Integer
Get
Return m_Salary
End Get
Set(ByVal value As Integer)
m_Salary = value
End Set
End Property
Public Sub New(ByVal first_name As String, ByVal last_name As String, ByVal student_id As Integer, ByVal new_department As String, ByVal new_office As Integer, ByVal new_extension As Integer, ByVal social_security_number As String, ByVal new_salary As Integer)
m_FirstName = first_name
m_LastName = last_name
m_EmployeeId = student_id
m_Department = new_department
m_Office = new_office
m_Extension = new_extension
m_SocialSecurityNumber = social_security_number
m_Salary = new_salary
End Sub
End Class
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Partial Public Class Form1
Inherits System.Windows.Forms.Form
'Form overrides dispose to clean up the component list.
<System.Diagnostics.DebuggerNonUserCode()> _
Protected Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> _
Private Sub InitializeComponent()
Me.PropertyGrid1 = New System.Windows.Forms.PropertyGrid
Me.SuspendLayout()
'
'PropertyGrid1
'
Me.PropertyGrid1.Dock = System.Windows.Forms.DockStyle.Fill
Me.PropertyGrid1.Location = New System.Drawing.Point(0, 0)
Me.PropertyGrid1.Name = "PropertyGrid1"
Me.PropertyGrid1.Size = New System.Drawing.Size(292, 273)
Me.PropertyGrid1.TabIndex = 0
'
'Form1
'
Me.AutoScaleDimensions = New System.Drawing.SizeF(6.0!, 13.0!)
Me.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font
Me.ClientSize = New System.Drawing.Size(292, 273)
Me.Controls.Add(Me.PropertyGrid1)
Me.Name = "Form1"
Me.Text = "Employee"
Me.ResumeLayout(False)
End Sub
Friend WithEvents PropertyGrid1 As System.Windows.Forms.PropertyGrid
End Class
Related examples in the same category