Using CheckBoxes to toggle italic and bold styles
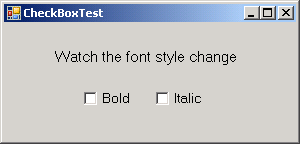
Imports System
Imports System.Drawing
Imports System.Windows.Forms
Public Class MainClass
Shared Sub Main()
Dim myform As Form = New FrmCheckBox()
Application.Run(myform)
End Sub ' Main
End Class
Public Class FrmCheckBox
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
Friend WithEvents chkItalic As System.Windows.Forms.CheckBox
Friend WithEvents lblOutput As System.Windows.Forms.Label
Friend WithEvents chkBold As System.Windows.Forms.CheckBox
'Required by the Windows Form Designer
Private components As System.ComponentModel.Container
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.lblOutput = New System.Windows.Forms.Label()
Me.chkItalic = New System.Windows.Forms.CheckBox()
Me.chkBold = New System.Windows.Forms.CheckBox()
Me.SuspendLayout()
'
'lblOutput
'
Me.lblOutput.Font = New System.Drawing.Font("Microsoft Sans Serif", 11.25!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.lblOutput.Location = New System.Drawing.Point(48, 24)
Me.lblOutput.Name = "lblOutput"
Me.lblOutput.Size = New System.Drawing.Size(208, 24)
Me.lblOutput.TabIndex = 0
Me.lblOutput.Text = "Watch the font style change"
'
'chkItalic
'
Me.chkItalic.Font = New System.Drawing.Font("Microsoft Sans Serif", 9.75!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.chkItalic.Location = New System.Drawing.Point(152, 64)
Me.chkItalic.Name = "chkItalic"
Me.chkItalic.Size = New System.Drawing.Size(64, 24)
Me.chkItalic.TabIndex = 2
Me.chkItalic.Text = "Italic"
'
'chkBold
'
Me.chkBold.Font = New System.Drawing.Font("Microsoft Sans Serif", 9.75!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.chkBold.Location = New System.Drawing.Point(80, 64)
Me.chkBold.Name = "chkBold"
Me.chkBold.Size = New System.Drawing.Size(56, 24)
Me.chkBold.TabIndex = 1
Me.chkBold.Text = "Bold"
'
'FrmCheckBox
'
Me.AutoScaleBaseSize = New System.Drawing.Size(6, 14)
Me.ClientSize = New System.Drawing.Size(292, 117)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.chkItalic, Me.chkBold, Me.lblOutput})
Me.Font = New System.Drawing.Font("Microsoft Sans Serif", 9!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.Name = "FrmCheckBox"
Me.Text = "CheckBoxTest"
Me.ResumeLayout(False)
End Sub
#End Region
' use Xor to toggle italic, keep other styles same
Private Sub chkItalic_CheckedChanged _
(ByVal sender As System.Object, ByVal e As System.EventArgs) _
Handles chkItalic.CheckedChanged
lblOutput.Font = New Font(lblOutput.Font.Name, _
lblOutput.Font.Size, lblOutput.Font.Style _
Xor FontStyle.Italic)
End Sub ' chkItalic_CheckedChanged
' use Xor to toggle bold, keep other styles same
Private Sub chkBold_CheckedChanged _
(ByVal sender As System.Object, ByVal e As System.EventArgs) _
Handles chkBold.CheckedChanged
lblOutput.Font = New Font(lblOutput.Font.Name, _
lblOutput.Font.Size, lblOutput.Font.Style _
Xor FontStyle.Bold)
End Sub
End Class
Related examples in the same category