Using a TreeView to display the directory structure
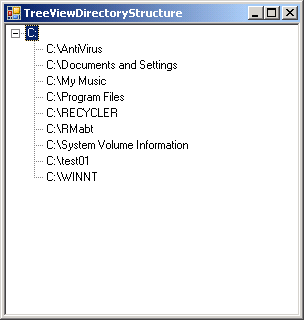
Imports System
Imports System.Drawing
Imports System.Windows.Forms
Imports System.IO
Public Class MainClass
Shared Sub Main()
Dim myform As Form = New FrmTreeViewDirectory()
Application.Run(myform)
End Sub ' Main
End Class
Public Class FrmTreeViewDirectory
Inherits Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent()
'call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If Disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(Disposing)
End Sub
' contains view of c: drive directory structure
Friend WithEvents treDirectory As System.Windows.Forms.TreeView
'Required by the Windows Form Designer
Private components As System.ComponentModel.Container
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.treDirectory = New System.Windows.Forms.TreeView()
Me.SuspendLayout()
'
'treDirectory
'
Me.treDirectory.Dock = System.Windows.Forms.DockStyle.Fill
Me.treDirectory.ImageIndex = -1
Me.treDirectory.Name = "treDirectory"
Me.treDirectory.SelectedImageIndex = -1
Me.treDirectory.Size = New System.Drawing.Size(296, 293)
Me.treDirectory.TabIndex = 0
'
'FrmTreeViewDirectory
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(296, 293)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.treDirectory})
Me.Name = "FrmTreeViewDirectory"
Me.Text = "TreeViewDirectoryStructure"
Me.ResumeLayout(False)
End Sub
#End Region
Public Sub PopulateTreeView(ByVal directoryValue As String, _
ByVal parentNode As TreeNode)
Try
Dim directoryArray As String() = Directory.GetDirectories(directoryValue)
If directoryArray.Length <> 0 Then
Dim currentDirectory As String
For Each currentDirectory In directoryArray
Dim myNode As TreeNode = New TreeNode(currentDirectory)
parentNode.Nodes.Add(myNode)
' PopulateTreeView(currentDirectory, myNode)
Next
End If
Catch unauthorized As UnauthorizedAccessException
parentNode.Nodes.Add("Access Denied")
End Try
End Sub
Private Sub FrmTreeViewDirectory_Load(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles MyBase.Load
treDirectory.Nodes.Add("C:")
PopulateTreeView("C:\", treDirectory.Nodes(0))
End Sub
End Class
Related examples in the same category