Array passed By Value
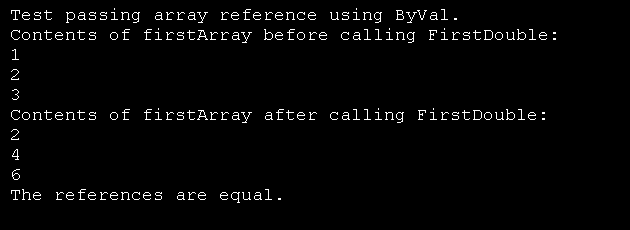
Imports System
Public Class MainClass
Shared Sub Main(ByVal args As String())
Dim i As Integer
Dim firstArray As Integer()
Dim firstArrayCopy As Integer()
firstArray = New Integer() {1, 2, 3}
firstArrayCopy = firstArray
Console.WriteLine("Test passing array reference using ByVal.")
Console.WriteLine("Contents of firstArray before calling FirstDouble: ")
For i = 0 To firstArray.GetUpperBound(0)
Console.WriteLine(firstArray(i))
Next
' pass firstArray using ByVal
FirstDouble(firstArray)
Console.WriteLine("Contents of firstArray after calling FirstDouble: ")
' print contents of firstArray
For i = 0 To firstArray.GetUpperBound(0)
Console.WriteLine(firstArray(i) & " ")
Next
' test whether reference was changed by FirstDouble
If firstArray Is firstArrayCopy Then
Console.WriteLine("The references are equal.")
Else
Console.WriteLine("The references are not equal.")
End If
End Sub
' procedure modifies elements of array and assigns
' new reference (note ByVal)
Shared Sub FirstDouble(ByVal array As Integer())
Dim i As Integer
' double each element value
For i = 0 To array.GetUpperBound(0)
array(i) *= 2
Next
' create new reference, assign it to array
array = New Integer() {11, 12, 13}
End Sub
End Class
Related examples in the same category