Define and use Attribute to track bugs
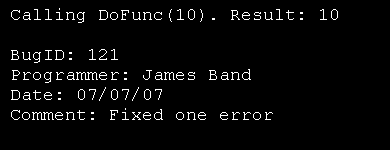
Imports System
Imports System.Reflection
Public Class MainClass
Shared Sub Main( )
Dim mm As New MyMath( )
Console.WriteLine("Calling DoFunc(10). Result: {0}", mm.DoFunc1(10))
Dim inf As System.Reflection.MemberInfo = GetType(MyMath)
Dim attributes( ) As Attribute
attributes = _
inf.GetCustomAttributes(GetType(BugFixAttribute), False)
Dim attribute As [Object]
For Each attribute In attributes
Dim bfa As BugFixAttribute = CType(attribute, BugFixAttribute)
Console.WriteLine(ControlChars.Lf + "BugID: {0}", bfa.BugID)
Console.WriteLine("Programmer: {0}", bfa.Programmer)
Console.WriteLine("Date: {0}", bfa.theDate)
Console.WriteLine("Comment: {0}", bfa.Comment)
Next attribute
End Sub
End Class
<AttributeUsage(AttributeTargets.Class Or _
AttributeTargets.Constructor Or _
AttributeTargets.Field Or _
AttributeTargets.Method Or _
AttributeTargets.Property, AllowMultiple:=True)> _
Public Class BugFixAttribute
Inherits System.Attribute
Private mBugID As Integer
Private mComment As String
Private mDate As String
Private mProgrammer As String
Public Sub New( _
ByVal bugID As Integer, _
ByVal programmer As String, _
ByVal theDate As String)
mBugID = bugID
mProgrammer = programmer
mDate = theDate
End Sub
Public ReadOnly Property BugID( ) As Integer
Get
Return mBugID
End Get
End Property
Public Property Comment( ) As String
Get
Return mComment
End Get
Set(ByVal Value As String)
mComment = Value
End Set
End Property
Public ReadOnly Property theDate( ) As String
Get
Return mDate
End Get
End Property
Public ReadOnly Property Programmer( ) As String
Get
Return mProgrammer
End Get
End Property
End Class
<BugFixAttribute(121, "James Band", "07/07/07", _
Comment:="Fixed one error")> _
Public Class MyMath
Public Function DoFunc1(ByVal param1 As Double) As Double
Return param1
End Function
End Class
Related examples in the same category