Pass Structure into a Function
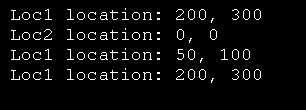
Imports System
Public Class MainClass
Shared Sub Main(ByVal args As String())
' create an instance of the structure
Dim loc1 As New Location(200, 300)
' display the values in the structure
Console.WriteLine("Loc1 location: {0}", loc1)
' invoke the default constructor
Dim loc2 As New Location( )
Console.WriteLine("Loc2 location: {0}", loc2)
' pass the structure to a method
myFunc(loc1)
' redisplay the values in the structure
Console.WriteLine("Loc1 location: {0}", loc1)
End Sub
' method takes a structure as a parameter
Shared Public Sub myFunc(ByVal loc As Location)
' modify the values through the properties
loc.XVal = 50
loc.YVal = 100
Console.WriteLine("Loc1 location: {0}", loc)
End Sub 'myFunc
End Class
Public Structure Location
' the Structure has private data
Private myXVal As Integer
Private myYVal As Integer
' constructor
Public Sub New( _
ByVal xCoordinate As Integer, ByVal yCoordinate As Integer)
myXVal = xCoordinate
myYVal = yCoordinate
End Sub 'New
' property
Public Property XVal( ) As Integer
Get
Return myXVal
End Get
Set(ByVal Value As Integer)
myXVal = Value
End Set
End Property
Public Property YVal( ) As Integer
Get
Return myYVal
End Get
Set(ByVal Value As Integer)
myYVal = Value
End Set
End Property
' Display the structure as a String
Public Overrides Function ToString( ) As String
Return [String].Format("{0}, {1}", xVal, yVal)
End Function 'ToString
End Structure 'Location
Related examples in the same category