Use Delegate to implement custome sort
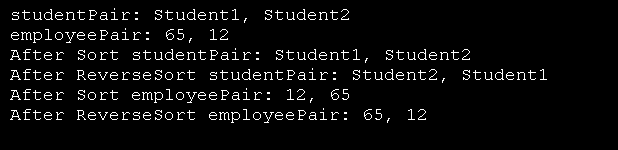
Imports System
Public Class MainClass
Shared Sub Main( )
Dim Student1 As New Student("Student1")
Dim Student2 As New Student("Student2")
Dim Employee1 As New Employee(65)
Dim Employee2 As New Employee(12)
Dim studentPair As New Pair(Student1, Student2)
Dim employeePair As New Pair(Employee1, Employee2)
Console.WriteLine("studentPair: {0}", _
studentPair.ToString( ))
Console.WriteLine("employeePair: {0}", _
employeePair.ToString( ))
Dim theStudentDelegate As New _
Pair.WhichIsSmaller(AddressOf Student.WhichStudentIsSmaller)
Dim theEmployeeDelegate As New _
Pair.WhichIsSmaller(AddressOf Employee.WhichEmployeeIsSmaller)
studentPair.Sort(theStudentDelegate)
Console.WriteLine("After Sort studentPair: {0}", _
studentPair.ToString( ))
studentPair.ReverseSort(theStudentDelegate)
Console.WriteLine("After ReverseSort studentPair: {0}", _
studentPair.ToString( ))
employeePair.Sort(theEmployeeDelegate)
Console.WriteLine("After Sort employeePair: {0}", _
employeePair.ToString( ))
employeePair.ReverseSort(theEmployeeDelegate)
Console.WriteLine("After ReverseSort employeePair: {0}", _
employeePair.ToString( ))
End Sub 'Main
End Class
Public Enum Comparison
theFirst = 1
theSecond = 2
End Enum
Public Class Pair
Private thePair(2) As Object
Public Delegate Function WhichIsSmaller(ByVal obj1 As Object, ByVal obj2 As Object) As Comparison
Public Sub New(ByVal firstObject As Object,ByVal secondObject As Object)
thePair(0) = firstObject
thePair(1) = secondObject
End Sub
Public Sub Sort(ByVal theDelegatedFunc As WhichIsSmaller)
If theDelegatedFunc(thePair(0), thePair(1)) = _
Comparison.theSecond Then
Dim temp As Object = thePair(0)
thePair(0) = thePair(1)
thePair(1) = temp
End If
End Sub
Public Sub ReverseSort(ByVal theDelegatedFunc As WhichIsSmaller)
If theDelegatedFunc(thePair(0), thePair(1)) = _
Comparison.theFirst Then
Dim temp As Object = thePair(0)
thePair(0) = thePair(1)
thePair(1) = temp
End If
End Sub
Public Overrides Function ToString( ) As String
Return thePair(0).ToString( ) & ", " & thePair(1).ToString( )
End Function
End Class
Public Class Employee
Private weight As Integer
Public Sub New(ByVal weight As Integer)
Me.weight = weight
End Sub
Public Shared Function WhichEmployeeIsSmaller(ByVal o1 As Object, ByVal o2 As Object) As Comparison
Dim d1 As Employee = DirectCast(o1, Employee)
Dim d2 As Employee = DirectCast(o2, Employee)
If d1.weight > d2.weight Then
Return Comparison.theSecond
Else
Return Comparison.theFirst
End If
End Function
Public Overrides Function ToString( ) As String
Return weight.ToString( )
End Function
End Class
Public Class Student
Private name As String
Public Sub New(ByVal name As String)
Me.name = name
End Sub
Public Shared Function WhichStudentIsSmaller( _
ByVal o1 As Object, ByVal o2 As Object) As Comparison
Dim s1 As Student = DirectCast(o1, Student)
Dim s2 As Student = DirectCast(o2, Student)
If String.Compare(s1.name, s2.name) < 0 Then
Return Comparison.theFirst
Else
Return Comparison.theSecond
End If
End Function
Public Overrides Function ToString( ) As String
Return name
End Function
End Class
Related examples in the same category