Shows multiple threads that print at different intervals
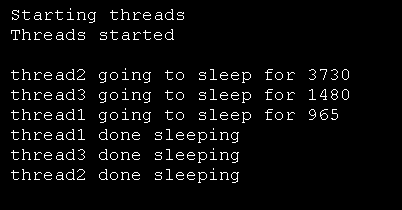
Imports System
Imports System.Threading
Public Class MainClass
Shared Sub Main()
Dim printer1 As New Messager()
Dim printer2 As New Messager()
Dim printer3 As New Messager()
Dim thread1 As New Thread(AddressOf printer1.Print)
Dim thread2 As New Thread(AddressOf printer2.Print)
Dim thread3 As New Thread(AddressOf printer3.Print)
thread1.Name = "thread1"
thread2.Name = "thread2"
thread3.Name = "thread3"
Console.WriteLine("Starting threads")
thread1.Start()
thread2.Start()
thread3.Start()
Console.WriteLine("Threads started" & vbCrLf)
End Sub ' Main
End Class
Public Class Messager
Private sleepTime As Integer
Private Shared randomObject As New Random()
Public Sub New()
sleepTime = randomObject.Next(5001)
End Sub ' New
Public Sub Print()
Dim current As Thread = Thread.CurrentThread
Console.WriteLine(current.Name & " going to sleep for " & _
sleepTime)
Thread.Sleep(sleepTime)
Console.WriteLine(current.Name & " done sleeping")
End Sub ' Print
End Class
Related examples in the same category