Create Binding for ListView in code
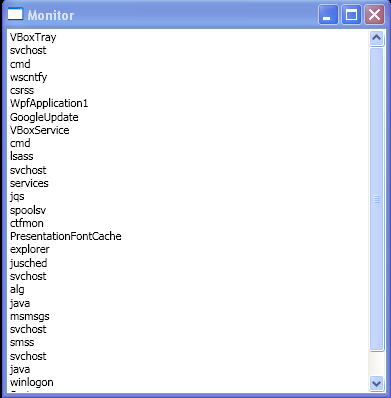
<Window x:Class="WpfApplication1.Monitor"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:diag="clr-namespace:System.Diagnostics;assembly=System"
xmlns:system="clr-namespace:System;assembly=mscorlib"
Title="Monitor" Height="400" Width="389">
<Grid>
<Grid.Resources>
<ObjectDataProvider x:Key="processes" MethodName="GetProcesses" ObjectType="{x:Type diag:Process}"/>
<ObjectDataProvider x:Key="dateinfo" ObjectType="{x:Type system:DateTime}"/>
</Grid.Resources>
<ListView Name="listView1" ItemsSource="{Binding Source={StaticResource processes}}">
</ListView>
</Grid>
</Window>
//File:Window.xaml.vb
Imports System
Imports System.Collections.Generic
Imports System.Linq
Imports System.Text
Imports System.Windows
Imports System.Windows.Controls
Imports System.Windows.Data
Imports System.Windows.Documents
Imports System.Windows.Input
Imports System.Windows.Media
Imports System.Windows.Media.Imaging
Imports System.Windows.Shapes
Imports System.Diagnostics
Imports System.Windows.Markup
Imports System.Xml
Imports System.IO
Imports System.Timers
Namespace WpfApplication1
Public Partial Class Monitor
Inherits Window
Public Sub New()
InitializeComponent()
Dim provider As New ObjectDataProvider()
provider.ObjectType = GetType(Process)
provider.MethodName = "GetProcesses"
Dim binding As New Binding()
PresentationTraceSources.SetTraceLevel(binding, PresentationTraceLevel.High)
binding.Source = provider
binding.Mode = BindingMode.OneWay
binding.UpdateSourceTrigger = UpdateSourceTrigger.Explicit
listView1.ItemTemplate = CreateItemTemplateFromXaml()
listView1.SetBinding(ListView.ItemsSourceProperty, binding)
End Sub
Private Function CreateItemTemplateFromXaml() As DataTemplate
Dim templateXml As String = "<DataTemplate xmlns='http://schemas.microsoft.com/winfx/2006/xaml/presentation' xmlns:x='http://schemas.microsoft.com/winfx/2006/xaml'><TextBlock Text='{Binding Path=ProcessName}'/></DataTemplate>"
Dim templateBytes As Byte() = Encoding.UTF8.GetBytes(templateXml)
Using templateStream As New MemoryStream(templateBytes)
Dim template As DataTemplate = DirectCast(XamlReader.Load(templateStream), DataTemplate)
Return template
End Using
End Function
Private Function CreateItemTemplate() As DataTemplate
Dim template As New DataTemplate()
Dim panelFactory As New FrameworkElementFactory(GetType(WrapPanel))
Dim idTextFactory As New FrameworkElementFactory(GetType(TextBlock))
idTextFactory.SetBinding(TextBlock.TextProperty, New Binding("Id"))
idTextFactory.SetValue(TextBlock.MinWidthProperty, 80.0)
Dim workingSetTextFactory As New FrameworkElementFactory(GetType(TextBlock))
workingSetTextFactory.SetBinding(TextBlock.TextProperty, New Binding("WorkingSet"))
panelFactory.AppendChild(idTextFactory)
panelFactory.AppendChild(workingSetTextFactory)
template.VisualTree = panelFactory
Return template
End Function
End Class
End Namespace
Related examples in the same category