Get all children from a Panel
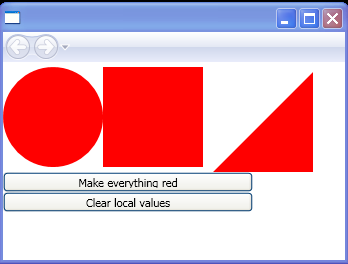
<StackPanel Name="root"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
x:Class="WpfApplication1.DPClearValue">
<StackPanel.Resources>
<Style TargetType="Button">
<Setter Property="Height" Value="20"/>
<Setter Property="Width" Value="250"/>
<Setter Property="HorizontalAlignment" Value="Left"/>
</Style>
<Style TargetType="Ellipse">
<Setter Property="Height" Value="50"/>
<Setter Property="Width" Value="50"/>
<Setter Property="Fill" Value="Black"/>
</Style>
<Style TargetType="Rectangle">
<Setter Property="Height" Value="50"/>
<Setter Property="Width" Value="50"/>
<Setter Property="Fill" Value="Blue"/>
</Style>
<Style TargetType="Polygon">
<Setter Property="Points" Value="10,60 60,60 60,10"/>
<Setter Property="Fill" Value="Blue"/>
</Style>
<Style x:Key="ShapeStyle" TargetType="Shape">
<Setter Property="Fill" Value="Red"/>
</Style>
</StackPanel.Resources>
<DockPanel Name="myDockPanel">
<Ellipse Height="100" Width="100" Style="{StaticResource ShapeStyle}"/>
<Rectangle Height="100" Width="100" Style="{StaticResource ShapeStyle}" />
<Polygon Points="10,110 110,110 110,10" Style="{StaticResource ShapeStyle}"/>
</DockPanel>
<Button Name="RedButton" Click="MakeEverythingRed">Make everything red</Button>
<Button Name="ClearButton" Click="RestoreDefaultProperties">
Clear local values
</Button>
</StackPanel>
//File:Window.xaml.vb
Imports System.Windows
Imports System.Collections
Imports System.Windows.Media
Imports System.Windows.Controls
Imports System.Windows.Shapes
Namespace WpfApplication1
Public Partial Class DPClearValue
Private Sub RestoreDefaultProperties(sender As Object, e As RoutedEventArgs)
Dim uic As UIElementCollection = myDockPanel.Children
For Each uie As Shape In uic
Dim locallySetProperties As LocalValueEnumerator = uie.GetLocalValueEnumerator()
While locallySetProperties.MoveNext()
Dim propertyToClear As DependencyProperty = DirectCast(locallySetProperties.Current.[Property], DependencyProperty)
If Not propertyToClear.[ReadOnly] Then
uie.ClearValue(propertyToClear)
End If
End While
Next
End Sub
Private Sub MakeEverythingRed(sender As Object, e As RoutedEventArgs)
Dim uic As UIElementCollection = myDockPanel.Children
For Each uie As Shape In uic
uie.Fill = New SolidColorBrush(Colors.Red)
Next
End Sub
End Class
End Namespace
Related examples in the same category