XmlDataProvider and XmlNamespaceMapping
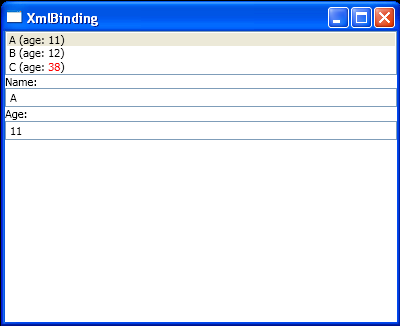
<Window x:Class="XmlBinding.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:XmlBinding"
Title="XmlBinding" Height="325" Width="400">
<Window.Resources>
<XmlDataProvider x:Key="Company" XPath="/sb:Company/sb:Employee">
<XmlDataProvider.XmlNamespaceManager>
<XmlNamespaceMappingCollection>
<XmlNamespaceMapping Uri="http://company.com" Prefix="sb" />
</XmlNamespaceMappingCollection>
</XmlDataProvider.XmlNamespaceManager>
<x:XData>
<Company xmlns="http://company.com">
<Employee Name="A" Age="11" />
<Employee Name="B" Age="12" />
<Employee Name="C" Age="38" />
</Company>
</x:XData>
</XmlDataProvider>
<local:AgeToForegroundConverter x:Key="ageConverter" />
</Window.Resources>
<StackPanel DataContext="{StaticResource Company}">
<ListBox ItemsSource="{Binding}" IsSynchronizedWithCurrentItem="True">
<ListBox.GroupStyle>
<x:Static Member="GroupStyle.Default" />
</ListBox.GroupStyle>
<ListBox.ItemTemplate>
<DataTemplate>
<TextBlock>
<TextBlock Text="{Binding XPath=@Name}" />
(age: <TextBlock Text="{Binding XPath=@Age}" Foreground="{Binding XPath=@Age, Converter={StaticResource ageConverter}}" />)
</TextBlock>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
<TextBlock>Name:</TextBlock>
<TextBox Text="{Binding XPath=@Name}" />
<TextBlock>Age:</TextBlock>
<TextBox Text="{Binding XPath=@Age}" Foreground="{Binding XPath=@Age, Converter={StaticResource ageConverter}}" />
</StackPanel>
</Window>
//File:Window.xaml.vb
Imports System
Imports System.Text
Imports System.Windows
Imports System.Windows.Controls
Imports System.Windows.Data
Imports System.Windows.Documents
Imports System.Windows.Input
Imports System.Windows.Media
Imports System.Windows.Media.Imaging
Imports System.Windows.Shapes
Imports System.Collections.Generic
Imports System.Diagnostics
Imports System.Collections
Imports System.Data
Imports System.Data.OleDb
Imports System.ComponentModel
Imports System.Xml
Namespace XmlBinding
Public Partial Class Window1
Inherits Window
Public Sub New()
InitializeComponent()
End Sub
Private Function GetCompanyView() As ICollectionView
Dim provider As DataSourceProvider = DirectCast(Me.FindResource("Company"), DataSourceProvider)
Return CollectionViewSource.GetDefaultView(provider.Data)
End Function
End Class
Public Class AgeToForegroundConverter
Implements IValueConverter
Public Function Convert(value As Object, targetType As Type, parameter As Object, culture As System.Globalization.CultureInfo) As Object Implements IValueConverter.Convert
Debug.Assert(targetType Is GetType(Brush))
Dim age As Integer = Integer.Parse(value.ToString())
Return (If(age > 25, Brushes.Red, Brushes.Black))
End Function
Public Function ConvertBack(value As Object, targetType As Type, parameter As Object, culture As System.Globalization.CultureInfo) As Object Implements IValueConverter.ConvertBack
Throw New NotImplementedException()
End Function
End Class
End Namespace
Related examples in the same category