using System;
using System.Drawing;
using System.Threading;
using System.Windows.Forms;
class Program : Form
{
private System.Windows.Forms.ProgressBar _ProgressBar;
[STAThread]
static void Main()
{
Application.Run(new Program());
}
public Program()
{
InitializeComponent();
ThreadStart threadStart = Increment;
threadStart.BeginInvoke(null, null);
}
void UpdateProgressBar()
{
if (_ProgressBar.InvokeRequired){
MethodInvoker updateProgressBar = UpdateProgressBar;
_ProgressBar.Invoke(updateProgressBar);
}else{
_ProgressBar.Increment(1);
}
}
private void Increment()
{
for (int i = 0; i < 100; i++)
{
UpdateProgressBar();
Thread.Sleep(100);
}
if (InvokeRequired)
{
Invoke(new MethodInvoker(Close));
}else{
Close();
}
}
private void InitializeComponent()
{
_ProgressBar = new ProgressBar();
SuspendLayout();
_ProgressBar.Location = new Point(13, 17);
_ProgressBar.Size = new Size(267, 19);
ClientSize = new Size(292, 53);
Controls.Add(this._ProgressBar);
Text = "Multithreading in Windows Forms";
ResumeLayout(false);
}
}
23.74.Thread UI |
| 23.74.1. | Mutex and UI | 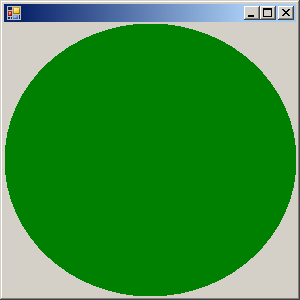 |
| 23.74.2. | Multithreading in Windows Forms |