/*******************************************************************************
* Copyright (c) 2000, 2004 IBM Corporation and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* IBM Corporation - initial API and implementation
*******************************************************************************/
/*
* BusyIndicator example snippet: display busy cursor during long running task
*
* For a list of all SWT example snippets see
* http://www.eclipse.org/swt/snippets/
*/
import org.eclipse.swt.SWT;
import org.eclipse.swt.custom.BusyIndicator;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.layout.GridData;
import org.eclipse.swt.layout.GridLayout;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Text;
public class BusyCursorDisplay {
public static void main(String[] args) {
final Display display = new Display();
final Shell shell = new Shell(display);
shell.setLayout(new GridLayout());
final Text text = new Text(shell, SWT.MULTI | SWT.BORDER | SWT.V_SCROLL);
text.setLayoutData(new GridData(GridData.FILL_BOTH));
final int[] nextId = new int[1];
Button b = new Button(shell, SWT.PUSH);
b.setText("invoke long running job");
b.addSelectionListener(new SelectionAdapter() {
public void widgetSelected(SelectionEvent e) {
Runnable longJob = new Runnable() {
boolean done = false;
int id;
public void run() {
Thread thread = new Thread(new Runnable() {
public void run() {
id = nextId[0]++;
display.syncExec(new Runnable() {
public void run() {
if (text.isDisposed())
return;
text.append("\nStart long running task " + id);
}
});
for (int i = 0; i < 100000; i++) {
if (display.isDisposed())
return;
System.out.println("do task that takes a long time in a separate thread " + id);
}
if (display.isDisposed())
return;
display.syncExec(new Runnable() {
public void run() {
if (text.isDisposed())
return;
text.append("\nCompleted long running task " + id);
}
});
done = true;
display.wake();
}
});
thread.start();
while (!done && !shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
}
};
BusyIndicator.showWhile(display, longJob);
}
});
shell.setSize(250, 150);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}
17.75.BusyIndicator |
| 17.75.1. | Using BusyIndicator | 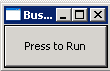 |
| 17.75.2. | BusyIndicator: display busy cursor during long running task |