Send out email
Description
The following code shows how to send out email.
Example
<?xml version="1.0" encoding="utf-8"?>
<!--
Copyright (c) 2012 Manning
See the file license.txt for copying permission.
-->
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<Button
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:gravity="center"
android:onClick="sendFeedback"
android:text="Send Feedback" />
</RelativeLayout>
Java code
/*******************************************************************************
* Copyright (c) 2012 Manning/*from w ww . ja v a 2 s. c o m*/
* See the file license.txt for copying permission.
******************************************************************************/
import android.content.Context;
import android.content.pm.PackageInfo;
import android.content.pm.PackageManager.NameNotFoundException;
import android.os.Build;
import android.telephony.TelephonyManager;
import android.content.Context;
import android.content.Intent;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
public void sendFeedback(View v) {
LaunchEmailUtil.launchEmailToIntent(this);
}
}
class EnvironmentInfoUtil {
public static String getApplicationInfo(Context context) {
return String.format("%s\n%s\n%s\n%s\n%s\n%s\n",
getCountry(context), getBrandInfo(), getModelInfo(),
getDeviceInfo(), getVersionInfo(context), getLocale(context));
}
public static String getCountry(Context context) {
TelephonyManager mTelephonyMgr = (TelephonyManager) context
.getSystemService(Context.TELEPHONY_SERVICE);
return String.format("Country: %s",
mTelephonyMgr.getNetworkCountryIso());
}
public static String getModelInfo() {
return String.format("Model: %s", Build.MODEL);
}
public static String getBrandInfo() {
return String.format("Brand: %s", Build.BRAND);
}
public static String getDeviceInfo() {
return String.format("Device: %s", Build.DEVICE);
}
public static String getLocale(Context context) {
return String.format("Locale: %s", context.getResources()
.getConfiguration().locale.getDisplayName());
}
public static String getVersionInfo(Context context) {
String version = null;
try {
PackageInfo info = context.getPackageManager().getPackageInfo(
context.getPackageName(), 0);
version = info.versionName + " (release " + info.versionCode
+ ")";
} catch (NameNotFoundException e) {
version = "not_found";
}
return String.format("Version: %s", version);
}
}
class LaunchEmailUtil {
public static void launchEmailToIntent(Context context) {
Intent msg = new Intent(Intent.ACTION_SEND);
StringBuilder body = new StringBuilder("\n\n----------\n");
body.append(EnvironmentInfoUtil.getApplicationInfo(context));
msg.putExtra(Intent.EXTRA_EMAIL,"feed@back.com".split(", "));
msg.putExtra(Intent.EXTRA_SUBJECT,"feedback_subject");
msg.putExtra(Intent.EXTRA_TEXT, body.toString());
msg.setType("message/rfc822");
context.startActivity(Intent.createChooser(msg,"send email"));
}
}
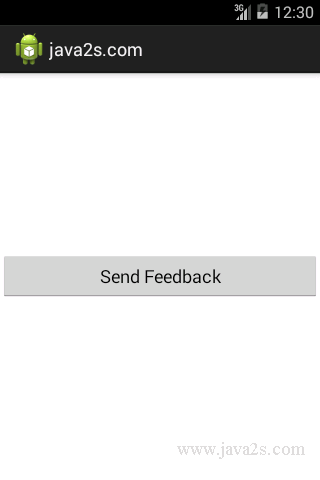