Translate coordinate system
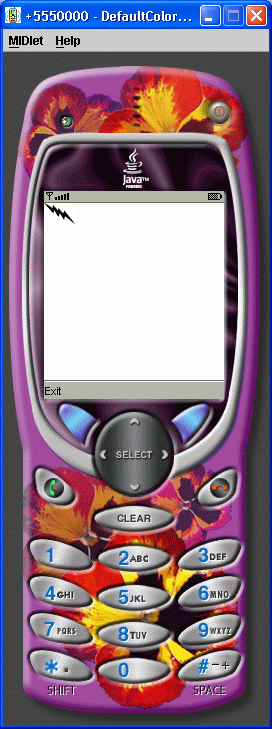
/*--------------------------------------------------
* Translate.java
*
* Translate coordinate system
*
* Example from the book: Core J2ME Technology
* Copyright John W. Muchow http://www.CoreJ2ME.com
* You may use/modify for any non-commercial purpose
*-------------------------------------------------*/
import javax.microedition.midlet.*;
import javax.microedition.lcdui.*;
public class Translate extends MIDlet
{
private Display display; // The display
private TranslateCanvas canvas; // Canvas
public Translate()
{
display = Display.getDisplay(this);
canvas = new TranslateCanvas(this);
}
protected void startApp()
{
display.setCurrent( canvas );
}
protected void pauseApp()
{ }
protected void destroyApp( boolean unconditional )
{ }
public void exitMIDlet()
{
destroyApp(true);
notifyDestroyed();
}
}
/*--------------------------------------------------
* Class Translate
*
* Draw image using translated coordinates
*-------------------------------------------------*/
class TranslateCanvas extends Canvas implements CommandListener
{
private Command cmExit; // Exit midlet
private Translate midlet;
private Image im = null;
private int translatex = 0, translatey = 0;
public TranslateCanvas(Translate midlet)
{
this.midlet = midlet;
// Create exit command & listen for events
cmExit = new Command("Exit", Command.EXIT, 1);
addCommand(cmExit);
setCommandListener(this);
try
{
// Create immutable image
im = Image.createImage("/bolt.png");
}
catch (java.io.IOException e)
{
System.err.println("Unable to locate or read .png file");
}
}
protected void paint(Graphics g)
{
if (im != null)
{
// Clear the background
g.setColor(255, 255, 255);
g.fillRect(0, 0, getWidth(), getHeight());
// Translate coordinates
g.translate(translatex, translatey);
// Always draw at 0,0
g.drawImage(im, 0, 0, Graphics.LEFT | Graphics.TOP);
}
}
public void commandAction(Command c, Displayable d)
{
if (c == cmExit)
midlet.exitMIDlet();
}
protected void keyPressed(int keyCode)
{
switch (getGameAction(keyCode))
{
case UP:
// If scrolling off the top, roll around to bottom
if (translatey - im.getHeight() < 0)
translatey = getHeight() - im.getHeight();
else
translatey -= im.getHeight();
break;
case DOWN:
// If scrolling off the bottom, roll around to top
if ((translatey + im.getHeight() + im.getHeight()) > getHeight())
translatey = 0;
else
translatey += im.getHeight();
break;
case LEFT:
// If scrolling off the left, bring around to right
if (translatex - im.getWidth() < 0)
translatex = getWidth() - im.getWidth();
else
translatex -= im.getWidth();
break;
case RIGHT:
// If scrolling off the right, bring around to left
if ((translatex + im.getWidth() + translatex) > getWidth())
translatex = 0;
else
translatex += im.getWidth();
break;
}
repaint();
}
}
Related examples in the same category