Draw a line for the ascent, descent, baseline, textOrigin
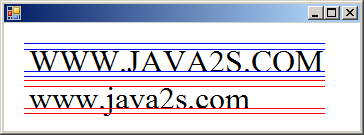
using System;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Drawing.Imaging;
public class Form1 : System.Windows.Forms.Form
{
public Form1()
{
InitializeComponent();
}
private void InitializeComponent()
{
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 273);
this.Text = "";
this.Resize += new System.EventHandler(this.Form1_Resize);
this.Paint += new System.Windows.Forms.PaintEventHandler(this.Form1_Paint);
}
static void Main()
{
Application.Run(new Form1());
}
private void Form1_Paint(object sender, System.Windows.Forms.PaintEventArgs e)
{
Graphics g = e.Graphics;
g.FillRectangle(Brushes.White, this.ClientRectangle);
FontFamily ff = new FontFamily("Times New Roman");
float emSizeInGU = 24f;
Font f = new Font(ff, emSizeInGU);
// Get the design unit metrics from the font family
int emSizeInDU = ff.GetEmHeight(FontStyle.Regular);
int ascentInDU = ff.GetCellAscent(FontStyle.Regular);
int descentInDU = ff.GetCellDescent(FontStyle.Regular);
int lineSpacingInDU = ff.GetLineSpacing(FontStyle.Regular);
// Calculate the GraphicsUnit metrics from the font
float ascentInGU = ascentInDU * (emSizeInGU / emSizeInDU);
float descentInGU = descentInDU * (emSizeInGU / emSizeInDU);
float lineSpacingInGU = lineSpacingInDU * (emSizeInGU / emSizeInDU);
// Draw two lines of the text string
PointF textOrigin = new PointF(20, 20);
PointF nextLineOrigin = new PointF(textOrigin.X,
textOrigin.Y + f.Height);
g.DrawString("WWW.JAVA2S.COM", f, Brushes.Black, textOrigin);
g.DrawString("www.java2s.com", f, Brushes.Black, nextLineOrigin);
// Draw a line at the textOrigin
int lineLen = 300;
g.DrawLine(Pens.Blue, textOrigin, new PointF(textOrigin.X + lineLen, textOrigin.Y));
g.DrawLine(Pens.Red, nextLineOrigin, new PointF(nextLineOrigin.X + lineLen, nextLineOrigin.Y));
// Draw a line at the baseline
PointF p = new PointF(textOrigin.X, textOrigin.Y + lineSpacingInGU);
g.DrawLine(Pens.Blue, p, new PointF(p.X + lineLen, p.Y));
p = new PointF(nextLineOrigin.X, nextLineOrigin.Y + lineSpacingInGU);
g.DrawLine(Pens.Red, p, new PointF(p.X + lineLen, p.Y));
// Draw a line at the top of the ascent
p = new PointF(textOrigin.X, textOrigin.Y + lineSpacingInGU - ascentInGU);
g.DrawLine(Pens.Blue, p, new PointF(p.X + lineLen, p.Y));
p = new PointF(nextLineOrigin.X, nextLineOrigin.Y + lineSpacingInGU - ascentInGU);
g.DrawLine(Pens.Red, p, new PointF(p.X + lineLen, p.Y));
// Draw a line at the bottom of the descent
p = new PointF(textOrigin.X, textOrigin.Y + lineSpacingInGU + descentInGU);
g.DrawLine(Pens.Blue, p, new PointF(p.X + lineLen, p.Y));
p = new PointF(nextLineOrigin.X, nextLineOrigin.Y + lineSpacingInGU + descentInGU);
g.DrawLine(Pens.Red, p, new PointF(p.X + lineLen, p.Y));
}
private void Form1_Resize(object sender, System.EventArgs e)
{
Invalidate();
}
}
Related examples in the same category