Fonts Class
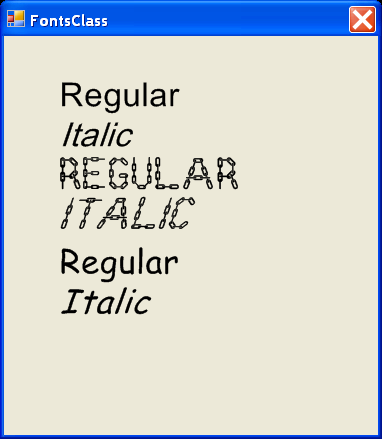
/*
GDI+ Programming in C# and VB .NET
by Nick Symmonds
Publisher: Apress
ISBN: 159059035X
*/
using System;
using System.Drawing;
using System.Drawing.Text;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace FontsClass_c
{
/// <summary>
/// Summary description for FontsClass.
/// </summary>
public class FontsClass : System.Windows.Forms.Form
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public FontsClass()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
//
// FontsClass
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 323);
this.MaximizeBox = false;
this.MinimizeBox = false;
this.Name = "FontsClass";
this.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen;
this.Text = "FontsClass";
this.Load += new System.EventHandler(this.FontsClass_Load);
}
#endregion
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new FontsClass());
}
private void FontsClass_Load(object sender, System.EventArgs e)
{
}
protected override void OnPaint(PaintEventArgs e)
{
Graphics G = e.Graphics;
G.TextRenderingHint=TextRenderingHint.AntiAlias;
int y = 0;
G.DrawString("Regular", Fonts.Arial_20, Brushes.Black, 50, y+=40);
G.DrawString("Italic", Fonts.ArialItalic_20, Brushes.Black, 50, y+=40);
G.DrawString("Regular", Fonts.Chain_20, Brushes.Black, 50, y+=40);
G.DrawString("Italic", Fonts.ChainItalic_20, Brushes.Black, 50, y+=40);
G.DrawString("Regular", Fonts.Comic_20, Brushes.Black, 50, y+=40);
G.DrawString("Italic", Fonts.ComicItalic_20, Brushes.Black, 50, y+=40);
}
}
public sealed class Fonts
{
private static PrivateFontCollection PFC;
private static FontFamily Arial_FF;
private static FontFamily Comic_FF;
private static FontFamily Chain_FF;
static Fonts(){
PFC = new PrivateFontCollection();
PFC.AddFontFile("C:\\WINNT\\Fonts\\Arial.ttf");
Arial_FF = PFC.Families[0];
PFC.AddFontFile("chainletters.ttf");
Chain_FF = PFC.Families[1];
PFC.AddFontFile("C:\\WINNT\\Fonts\\comic.ttf");
Comic_FF = PFC.Families[2];
}
public static FontFamily[] Families
{
get{ return PFC.Families; }
}
#region Arial Font
public static Font Arial_20
{
get {return new Font(Arial_FF, 20, FontStyle.Regular, GraphicsUnit.Point);}
}
public static Font ArialItalic_20
{
get {return new Font(Arial_FF, 20, FontStyle.Italic, GraphicsUnit.Point);}
}
#endregion
#region Chain font
public static Font Chain_20
{
get {return new Font(Chain_FF, 20, FontStyle.Regular, GraphicsUnit.Point);}
}
public static Font ChainItalic_20
{
get {return new Font(Chain_FF, 20, FontStyle.Italic, GraphicsUnit.Point);}
}
#endregion
#region Comic Font
public static Font Comic_20
{
get {return new Font(Comic_FF, 20, FontStyle.Regular, GraphicsUnit.Point);}
}
public static Font ComicItalic_20
{
get {return new Font(Comic_FF, 20, FontStyle.Italic, GraphicsUnit.Point);}
}
#endregion
}
}
FontsClass-c.zip( 32 k)Related examples in the same category