Get font from Font dialog and redraw string
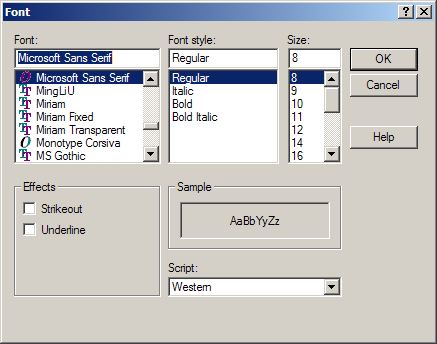
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
public class Form1 : System.Windows.Forms.Form
{
private System.ComponentModel.Container components;
private System.Windows.Forms.FontDialog fontDlg;
private Font currFont;
public Form1()
{
InitializeComponent();
CenterToScreen();
fontDlg = new System.Windows.Forms.FontDialog();
fontDlg.ShowHelp = true;
Text = "Click on me to change the font";
currFont = new Font("Times New Roman", 12);
}
private void InitializeComponent()
{
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 273);
this.Text = "Form1";
this.MouseUp += new System.Windows.Forms.MouseEventHandler(this.Form1_MouseUp);
this.Paint += new System.Windows.Forms.PaintEventHandler(this.Form1_Paint);
}
static void Main()
{
Application.Run(new Form1());
}
private void Form1_Paint(object sender, System.Windows.Forms.PaintEventArgs e)
{
Graphics g = e.Graphics;
g.DrawString("www.java2s.com...", currFont,
new SolidBrush(Color.Black), 0, 0);
}
private void Form1_MouseUp(object sender, System.Windows.Forms.MouseEventArgs e)
{
if (fontDlg.ShowDialog() != DialogResult.Cancel)
{
currFont = fontDlg.Font;
Invalidate();
}
}
}
Related examples in the same category