Reset the transformation
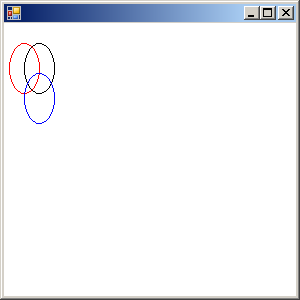
using System;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Drawing.Imaging;
public class Form1 : System.Windows.Forms.Form
{
public Form1()
{
InitializeComponent();
}
private void InitializeComponent()
{
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 273);
this.Text = "";
this.Resize += new System.EventHandler(this.Form1_Resize);
this.Paint += new System.Windows.Forms.PaintEventHandler(this.Form1_Paint);
}
static void Main()
{
Application.Run(new Form1());
}
private void Form1_Paint(object sender, System.Windows.Forms.PaintEventArgs e)
{
Graphics g = e.Graphics;
g.FillRectangle(Brushes.White, ClientRectangle);
g.DrawEllipse(Pens.Black, 20, 20, 30, 50);
// Translate by -15 pixels in horizontal direction
g.TranslateTransform(-15, 0);
g.DrawEllipse(Pens.Red, 20, 20, 30, 50);
// Reset the transformation
// Translate by 30 pixels in vertical direction
g.ResetTransform();
g.TranslateTransform(0, 30);
g.DrawEllipse(Pens.Blue, 20, 20, 30, 50);
}
private void Form1_Resize(object sender, System.EventArgs e)
{
Invalidate();
}
}
Related examples in the same category