Rotate Transform
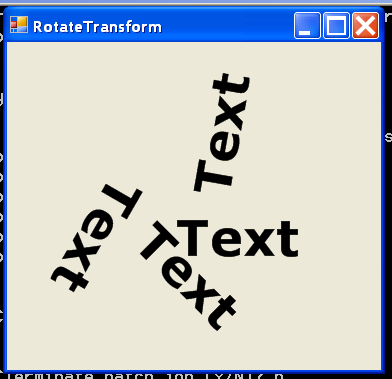
/*
User Interfaces in C#: Windows Forms and Custom Controls
by Matthew MacDonald
Publisher: Apress
ISBN: 1590590457
*/
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Drawing.Text;
namespace GDI_Basics
{
/// <summary>
/// Summary description for RotateTransform.
/// </summary>
public class RotateTransform : System.Windows.Forms.Form
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public RotateTransform()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if(components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
//
// RotateTransform
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 266);
this.Name = "RotateTransform";
this.Text = "RotateTransform";
this.Paint += new System.Windows.Forms.PaintEventHandler(this.RotateTransform_Paint);
}
#endregion
[STAThread]
static void Main()
{
Application.Run(new RotateTransform());
}
private void RotateTransform_Paint(object sender, System.Windows.Forms.PaintEventArgs e)
{
// Optimize text quality.
e.Graphics.TextRenderingHint = TextRenderingHint.AntiAliasGridFit;
// Move origin to center of form so we can rotate around that.
e.Graphics.TranslateTransform(this.Width / 2 - 30, this.Height / 2 - 30);
DrawText(e.Graphics);
e.Graphics.RotateTransform(45);
DrawText(e.Graphics);
e.Graphics.RotateTransform(75);
DrawText(e.Graphics);
e.Graphics.RotateTransform(160);
DrawText(e.Graphics);
}
private void DrawText(Graphics g)
{
g.DrawString("Text", new Font("Verdana", 30, FontStyle.Bold),
Brushes.Black, 0, 10);
}
}
}
Related examples in the same category