Solid Texture Brush
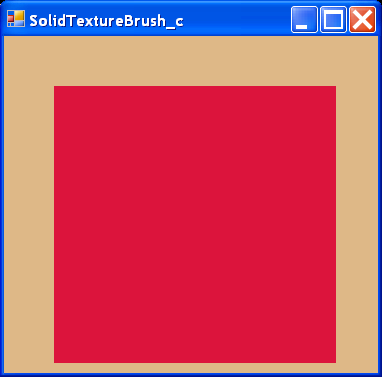
/*
GDI+ Programming in C# and VB .NET
by Nick Symmonds
Publisher: Apress
ISBN: 159059035X
*/
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace SolidTextureBrush_c
{
/// <summary>
/// Summary description for SolidTextureBrush_c.
/// </summary>
public class SolidTextureBrush_c : System.Windows.Forms.Form
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public SolidTextureBrush_c()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
//
// SolidTextureBrush_c
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 273);
this.Name = "SolidTextureBrush_c";
this.Text = "SolidTextureBrush_c";
this.Load += new System.EventHandler(this.SolidTextureBrush_c_Load);
}
#endregion
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new SolidTextureBrush_c());
}
private void SolidTextureBrush_c_Load(object sender, System.EventArgs e)
{
}
protected override void OnPaint(PaintEventArgs e)
{
Graphics G = e.Graphics;
//Brushes class
G.Clear(Color.BurlyWood);
Rectangle r = new Rectangle(new Point(50, 50),
new Size((int)(this.Width - 100), (int)(this.Height - 100)));
Brush b = Brushes.Crimson;
G.FillRectangle(b, r);
G.FillRectangle(Brushes.Crimson, r);
b.Dispose();
}
}
}
Related examples in the same category