Texture Brushes
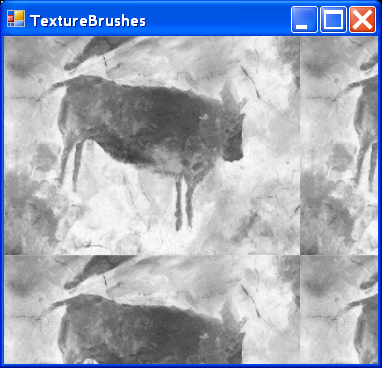
/*
User Interfaces in C#: Windows Forms and Custom Controls
by Matthew MacDonald
Publisher: Apress
ISBN: 1590590457
*/
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
namespace GDI_Basics
{
/// <summary>
/// Summary description for TextureBrushes.
/// </summary>
public class TextureBrushes : System.Windows.Forms.Form
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public TextureBrushes()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if(components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
//
// TextureBrushes
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 266);
this.Name = "TextureBrushes";
this.Text = "TextureBrushes";
this.Paint += new System.Windows.Forms.PaintEventHandler(this.TextureBrushes_Paint);
}
#endregion
private void TextureBrushes_Paint(object sender, System.Windows.Forms.PaintEventArgs e)
{
TextureBrush myBrush = new TextureBrush(Image.FromFile("tile.bmp"));
e.Graphics.FillRectangle(myBrush, e.Graphics.ClipBounds);
}
[STAThread]
static void Main()
{
Application.Run(new TextureBrushes());
}
}
}
Related examples in the same category