Define properties for class
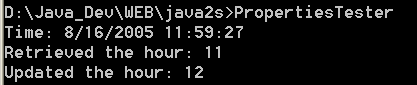
/*
Learning C#
by Jesse Liberty
Publisher: O'Reilly
ISBN: 0596003765
*/
using System;
namespace Properties
{
class Time
{
// private member variables
private int year;
private int month;
private int date;
private int hour;
private int minute;
private int second;
// create a property
public int Hour
{
get
{
return hour;
}
set
{
hour = value;
}
}
// public accessor methods
public void DisplayCurrentTime()
{
System.Console.WriteLine(
"Time: {0}/{1}/{2} {3}:{4}:{5}",
month, date, year, hour, minute, second);
}
// constructors
public Time(System.DateTime dt)
{
year = dt.Year;
month = dt.Month;
date = dt.Day;
hour = dt.Hour;
minute = dt.Minute;
second = dt.Second;
}
}
public class PropertiesTester
{
public void Run()
{
System.DateTime currentTime = System.DateTime.Now;
Time t = new Time(currentTime);
t.DisplayCurrentTime();
// access the hour to a local variable
int theHour = t.Hour;
// display it
System.Console.WriteLine("Retrieved the hour: {0}",
theHour);
// increment it
theHour++;
// reassign the incremented value back through
// the property
t.Hour = theHour;
// display the property
System.Console.WriteLine("Updated the hour: {0}", t.Hour);
}
[STAThread]
static void Main()
{
PropertiesTester t = new PropertiesTester();
t.Run();
}
}
}
Related examples in the same category