Demonstrates the use of properties to control how values are saved in fields
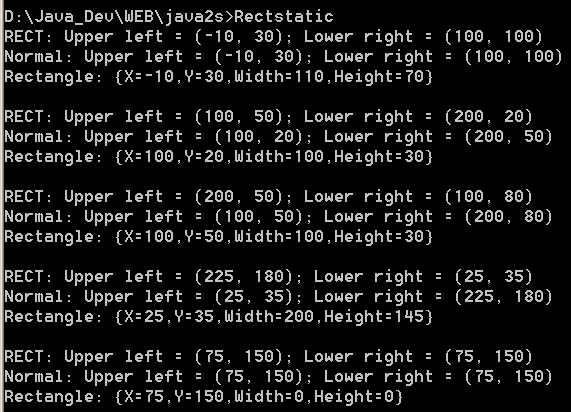
/*
C# Programming Tips & Techniques
by Charles Wright, Kris Jamsa
Publisher: Osborne/McGraw-Hill (December 28, 2001)
ISBN: 0072193794
*/
// Rect.cs - Demonstrates the use of properties to control how values are
// saved in fields
//
// This is a Visual Studio project. To compile outside of Visual
// Studio, use the following command line:
// C:>csc rect.cs
//
using System;
using System.Drawing;
namespace nsRect
{
struct POINT
{
public POINT (int x, int y)
{
this.cx = x;
this.cy = y;
}
public int cx;
public int cy;
public override string ToString ()
{
return (String.Format ("({0}, {1})", cx, cy));
}
}
struct RECT
{
public RECT (Rectangle rc)
{
m_UpperLeft.cx = rc.X;
m_UpperLeft.cy = rc.Y;
m_LowerRight.cx = rc.X + rc.Width;
m_LowerRight.cy = rc.Y + rc.Height;
}
// Define constructors
public RECT (POINT pt1, POINT pt2)
{
m_UpperLeft = pt1;
m_LowerRight = pt2;
}
public RECT (int x1, int y1, int x2, int y2)
{
m_UpperLeft.cx = x1;
m_UpperLeft.cy = y1;
m_LowerRight.cx = x2;
m_LowerRight.cy = y2;
}
public RECT (POINT pt1, int Width, int Height)
{
m_UpperLeft.cx = pt1.cx;
m_UpperLeft.cy = pt1.cy;
m_LowerRight.cx = pt1.cx + Width;
m_LowerRight.cy = pt1.cy + Height;
}
// Property to get and set the upper left point
public POINT UpperLeft
{
get {return (m_UpperLeft);}
set {m_UpperLeft = value;}
}
// Property to get and set the lower right point
public POINT LowerRight
{
get {return (m_LowerRight);}
set {m_LowerRight = value;}
}
// Property to return a normalized System.Drawing.ectangle object
public System.Drawing.Rectangle Rectangle
{
get
{
RECT rc = Normal;
return (new Rectangle (rc.UpperLeft.cx, rc.UpperLeft.cy,
rc.LowerRight.cx - rc.UpperLeft.cx,
rc.LowerRight.cy - rc.UpperLeft.cy));
}
}
// Property to return a normalized copy of this rectangle
public RECT Normal
{
get
{
return (new RECT (
Math.Min (m_LowerRight.cx, m_UpperLeft.cx),
Math.Min (m_LowerRight.cy, m_UpperLeft.cy),
Math.Max (m_LowerRight.cx, m_UpperLeft.cx),
Math.Max (m_LowerRight.cy, m_UpperLeft.cy))
);
}
}
private POINT m_UpperLeft;
private POINT m_LowerRight;
public override string ToString()
{
return (String.Format ("Upper left = {0}; Lower right = {1}",
m_UpperLeft, m_LowerRight));
}
}
public class Rect
{
static public void Main ()
{
// Define a "normal" rectangle
POINT pt1 = new POINT (-10,30);
POINT pt2 = new POINT (100, 100);
RECT rc = new RECT (pt1, pt2);
Console.WriteLine ("RECT: " + rc);
Console.WriteLine ("Normal: " + rc.Normal);
Console.WriteLine ("Rectangle: " + rc.Rectangle + "\n");
// Define a rectangle with normal x but not y
pt1.cx = 100;
pt1.cy = 50;
pt2.cx = 200;
pt2.cy = 20;
rc.UpperLeft = pt1;
rc.LowerRight = pt2;
Console.WriteLine ("RECT: " + rc);
Console.WriteLine ("Normal: " + rc.Normal);
Console.WriteLine ("Rectangle: " + rc.Rectangle + "\n");
// Define a rectangle with normal y but not x
pt1.cx = 200;
pt1.cy = 50;
pt2.cx = 100;
pt2.cy = 80;
rc.UpperLeft = pt1;
rc.LowerRight = pt2;
Console.WriteLine ("RECT: " + rc);
Console.WriteLine ("Normal: " + rc.Normal);
Console.WriteLine ("Rectangle: " + rc.Rectangle + "\n");
// Define a rectangle with both values of upper left greater than the lower y
pt1.cx = 225;
pt1.cy = 180;
pt2.cx = 25;
pt2.cy = 35;
rc.UpperLeft = pt1;
rc.LowerRight = pt2;
Console.WriteLine ("RECT: " + rc);
Console.WriteLine ("Normal: " + rc.Normal);
Console.WriteLine ("Rectangle: " + rc.Rectangle + "\n");
// Define a rectangle with points equal
pt1.cx = 75;
pt1.cy = 150;
pt2.cx = 75;
pt2.cy = 150;
rc.UpperLeft = pt1;
rc.LowerRight = pt2;
Console.WriteLine ("RECT: " + rc);
Console.WriteLine ("Normal: " + rc.Normal);
Console.WriteLine ("Rectangle: " + rc.Rectangle + "\n");
}
}
}
Related examples in the same category