Static class variable
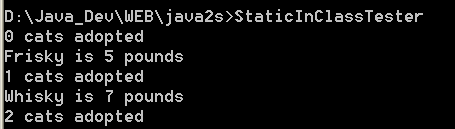
/*
Learning C#
by Jesse Liberty
Publisher: O'Reilly
ISBN: 0596003765
*/
using System;
namespace Test_Console_App_3
{
// declare a Cat class
// stripped down
class Cat
{
// a private static member to keep
// track of how many Cat objects have
// been created
private static int instances = 0;
private int weight;
private String name;
// cat constructor
// increments the count of Cats
public Cat(String name, int weight)
{
instances++;
this.name = name;
this.weight = weight;
}
// Static method to retrieve
// the current number of Cats
public static void HowManyCats()
{
Console.WriteLine("{0} cats adopted",
instances);
}
public void TellWeight()
{
Console.WriteLine("{0} is {1} pounds",
name, weight);
}
}
public class StaticInClassTester
{
public void Run()
{
Cat.HowManyCats();
Cat frisky = new Cat("Frisky", 5);
frisky.TellWeight();
Cat.HowManyCats();
Cat whiskers = new Cat("Whisky", 7);
whiskers.TellWeight();
Cat.HowManyCats();
}
static void Main()
{
StaticInClassTester t = new StaticInClassTester();
t.Run();
}
}
}
Related examples in the same category