illustrates the use of an ArrayList that contains objects of the Car class
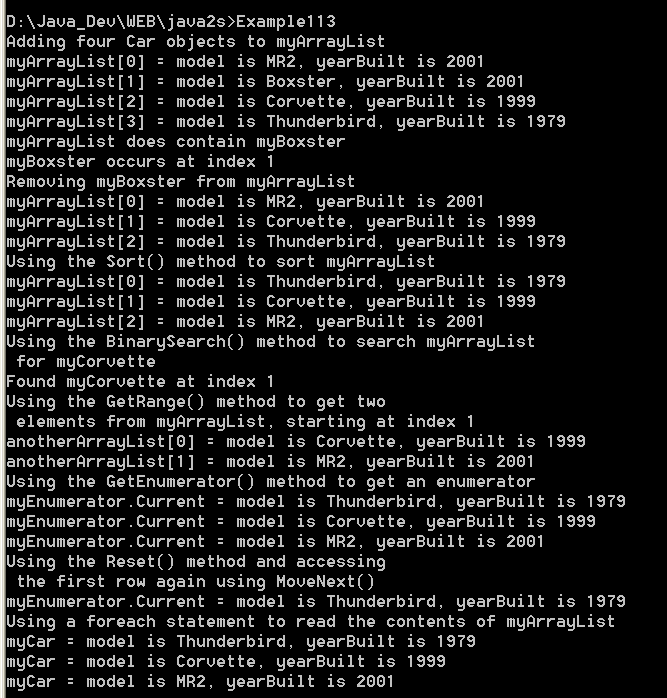
/*
Mastering Visual C# .NET
by Jason Price, Mike Gunderloy
Publisher: Sybex;
ISBN: 0782129110
*/
/*
Example11_3.cs illustrates the use of an ArrayList that contains
objects of the Car class
*/
using System;
using System.Collections;
// declare the Car class
class Car : IComparable
{
// declare the fields
public string model;
public int yearBuilt;
// define the constructor
public Car(string model, int yearBuilt)
{
this.model = model;
this.yearBuilt = yearBuilt;
}
// override the ToString() method
public override string ToString()
{
return "model is " + model + ", yearBuilt is " + yearBuilt;
}
// implement the Compare() method of IComparer
public int Compare(object lhs, object rhs)
{
Car lhsCar = (Car) lhs;
Car rhsCar = (Car) rhs;
if (lhsCar.yearBuilt < rhsCar.yearBuilt)
{
return -1;
}
else if (lhsCar.yearBuilt > rhsCar.yearBuilt)
{
return 1;
}
else
{
return 0;
}
}
// implement the CompareTo() method of IComparable
public int CompareTo(object rhs)
{
return Compare(this, rhs);
}
// alternative CompareTo() method that simply calls the
// CompareTo() method that comes with the int type
// (currently commented out)
/* public int CompareTo(object rhs)
{
Car rhsCar = (Car) rhs;
return this.yearBuilt.CompareTo(rhsCar.yearBuilt);
}*/
}
public class Example11_3
{
// the DisplayArrayList() method displays the elements in the
// supplied ArrayList
public static void DisplayArrayList(
string arrayListName, ArrayList myArrayList
)
{
for (int counter = 0; counter < myArrayList.Count; counter++)
{
Console.WriteLine(arrayListName + "[" + counter + "] = " +
myArrayList[counter]);
}
}
public static void Main()
{
// create an ArrayList object
ArrayList myArrayList = new ArrayList();
// add four Car objects to myArrayList using the Add() method
Console.WriteLine("Adding four Car objects to myArrayList");
Car myMR2 = new Car("MR2", 2001);
Car myBoxster = new Car("Boxster", 2001);
Car myCorvette = new Car("Corvette", 1999);
Car myThunderbird = new Car("Thunderbird", 1979);
myArrayList.Add(myMR2);
myArrayList.Add(myBoxster);
myArrayList.Add(myCorvette);
myArrayList.Add(myThunderbird);
DisplayArrayList("myArrayList", myArrayList);
// use the Contains() method to determine if myBoxster
// is in the ArrayList; if it is, then use the IndexOf()
// method to display the index
if (myArrayList.Contains(myBoxster))
{
Console.WriteLine("myArrayList does contain myBoxster");
int index = myArrayList.IndexOf(myBoxster);
Console.WriteLine("myBoxster occurs at index " + index);
}
// remove myBoxster from myArrayList
Console.WriteLine("Removing myBoxster from myArrayList");
myArrayList.Remove(myBoxster);
DisplayArrayList("myArrayList", myArrayList);
// use the Sort() method to sort myArrayList
Console.WriteLine("Using the Sort() method to sort myArrayList");
myArrayList.Sort();
DisplayArrayList("myArrayList", myArrayList);
// use the BinarySearch() method to search myArrayList for
// myCorvette
Console.WriteLine("Using the BinarySearch() method to search myArrayList\n" +
" for myCorvette");
int index2 = myArrayList.BinarySearch(myCorvette);
Console.WriteLine("Found myCorvette at index " + index2);
// use the GetRange() method to get a range of elements
// from myArrayList
Console.WriteLine("Using the GetRange() method to get two\n" +
" elements from myArrayList, starting at index 1");
ArrayList anotherArrayList = myArrayList.GetRange(1, 2);
DisplayArrayList("anotherArrayList", anotherArrayList);
// get an enumerator using the GetEnumerator() method
// and use it to read the elements in myArrayList
Console.WriteLine("Using the GetEnumerator() method to get an enumerator");
IEnumerator myEnumerator = myArrayList.GetEnumerator();
while (myEnumerator.MoveNext())
{
Console.WriteLine("myEnumerator.Current = " + myEnumerator.Current);
}
// use the Reset() method and access the first row again using MoveNext()
Console.WriteLine("Using the Reset() method and accessing\n" +
" the first row again using MoveNext()");
myEnumerator.Reset();
myEnumerator.MoveNext();
Console.WriteLine("myEnumerator.Current = " + myEnumerator.Current);
// Use a foreach statement to read the contents of myArrayList
Console.WriteLine("Using a foreach statement to read the contents of myArrayList");
foreach (Car myCar in myArrayList)
{
System.Console.WriteLine("myCar = " + myCar);
}
}
}
Related examples in the same category