Print a truth table for the logical operators
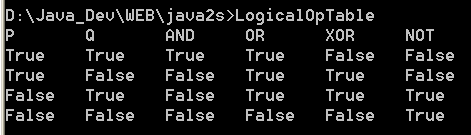
/*
C# A Beginner's Guide
By Schildt
Publisher: Osborne McGraw-Hill
ISBN: 0072133295
*/
/*
Project 2-2
Print a truth table for the logical operators.
*/
using System;
public class LogicalOpTable {
public static void Main() {
bool p, q;
Console.WriteLine("P\tQ\tAND\tOR\tXOR\tNOT");
p = true; q = true;
Console.Write(p + "\t" + q +"\t");
Console.Write((p&q) + "\t" + (p|q) + "\t");
Console.WriteLine((p^q) + "\t" + (!p));
p = true; q = false;
Console.Write(p + "\t" + q +"\t");
Console.Write((p&q) + "\t" + (p|q) + "\t");
Console.WriteLine((p^q) + "\t" + (!p));
p = false; q = true;
Console.Write(p + "\t" + q +"\t");
Console.Write((p&q) + "\t" + (p|q) + "\t");
Console.WriteLine((p^q) + "\t" + (!p));
p = false; q = false;
Console.Write(p + "\t" + q +"\t");
Console.Write((p&q) + "\t" + (p|q) + "\t");
Console.WriteLine((p^q) + "\t" + (!p));
}
}
Related examples in the same category