Talking to Mars: double value calculation

/*
C# A Beginner's Guide
By Schildt
Publisher: Osborne McGraw-Hill
ISBN: 0072133295
*/
/*
Project 2-1
Talking to Mars
Call this file mars.cs
*/
using System;
public class Mars {
public static void Main() {
double distance;
double lightspeed;
double delay;
double delay_in_min;
distance = 34000000; // 34,000,000 miles
lightspeed = 186000; // 186,000 per second
delay = distance / lightspeed;
Console.WriteLine("Time delay when talking to Mars: " +
delay + " seconds.");
delay_in_min = delay / 60;
Console.WriteLine("This is " + delay_in_min +
" minutes.");
}
}
Related examples in the same category
1. | Compute the area of a circle | |  |
2. | the differences
between int and double | |  |
3. | Implement the Pythagorean Theorem | | 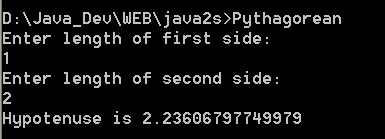 |
4. | converts Fahrenheit to Celsius | |  |
5. | Epsilon, PositiveInfinity, NegativeInfinity, MaxValue, MinValue | | |
6. | double number format: 0:C, 0:D9, 0:E, 0:F3, 0:N, 0:X, 0:x | | |
7. | Format double value | | |
8. | Get Decimal Places | | |
9. | Automatic conversion from double to string | | |
10. | An int, a short, a float, and a double are added together giving a double result. | | |
11. | Test to see if a double is a finite number (is not NaN or Infinity). | | |
12. | Double.Epsilon Field represents the smallest positive Double value that is greater than zero. | | |
13. | Availible Double Range | | |
14. | Truncates the specified double. | | |
15. | Is Nearly Equal | | |
16. | Shifts the given value into the range | | |