Demonstrates using the System.Timers.Timer class 2
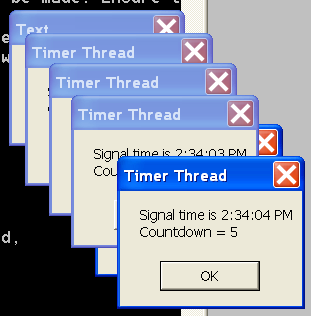
/*
C# Programming Tips & Techniques
by Charles Wright, Kris Jamsa
Publisher: Osborne/McGraw-Hill (December 28, 2001)
ISBN: 0072193794
*/
// Timer.cs -- demonstrates using the System.Timers.Timer class.
//
// Compile this program with the following command line:
// C:>csc Timer.cs
using System;
using System.Windows.Forms;
using System.Timers;
namespace nsDelegates
{
public class TimerAndDialog
{
static int countdown = 10;
static System.Timers.Timer timer;
static public void Main ()
{
// Create the timer object.
timer = new System.Timers.Timer (1000);
// Make it repeat. Setting this to false will cause just one event.
timer.AutoReset = true;
// Assign the delegate method.
timer.Elapsed += new ElapsedEventHandler(ProcessTimerEvent);
// Start the timer.
timer.Start ();
// Just wait.
MessageBox.Show ("Waiting for countdown", "Text");
}
// Method assigned to the timer delegate.
private static void ProcessTimerEvent (Object obj, ElapsedEventArgs e)
{
--countdown;
// If countdown has reached 0, it's time to exit.
if (countdown == 0)
{
timer.Close();
Environment.Exit (0);
}
// Make a string for a new message box.
string sigtime = e.SignalTime.ToString ();
string str = "Signal time is " + sigtime.Substring (sigtime.IndexOf(" ") + 1);
str += "\r\nCountdown = " + countdown;
// Show a message box.
MessageBox.Show (str, "Timer Thread");
}
}
}
Related examples in the same category