Get all methods from a class
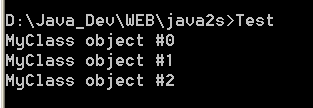
using System;
using System.Reflection;
public class Test
{
public static void Main(string[] args)
{
TheType.MyClass aClass = new TheType.MyClass();
Type t = aClass.GetType();
MethodInfo[] mi = t.GetMethods();
foreach(MethodInfo m in mi)
Console.WriteLine("Method: {0}", m.Name);
}
}
namespace TheType {
public interface IFaceOne {
void MethodA();
}
public interface IFaceTwo {
void MethodB();
}
public class MyClass: IFaceOne, IFaceTwo {
public int myIntField;
public string myStringField;
private double myDoubleField = 0;
public double getMyDouble(){
return myDoubleField;
}
public void myMethod(int p1, string p2)
{
}
public int MyProp
{
get { return myIntField; }
set { myIntField = value; }
}
public void MethodA() {}
public void MethodB() {}
}
}
Related examples in the same category