Catch Mouse Click event inside a strange shape
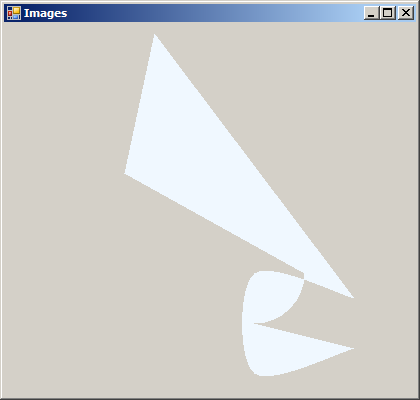
using System;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
public class Form1 : System.Windows.Forms.Form
{
GraphicsPath myPath = new GraphicsPath();
private bool isImageClicked = false;
public Form1()
{
InitializeComponent();
myPath.StartFigure();
myPath.AddLine(new Point(150, 10), new Point(120, 150));
myPath.AddArc(200, 200, 100, 100, 0, 90);
Point[] points = {new Point(350, 325), new Point(250, 350), new Point(250, 250), new Point(350, 275)};
myPath.AddCurve(points);
myPath.CloseFigure();
CenterToScreen();
}
private void InitializeComponent()
{
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 273);
this.Text = "Form1";
this.MouseUp += new System.Windows.Forms.MouseEventHandler(this.Form1_MouseUp);
this.Paint += new System.Windows.Forms.PaintEventHandler(this.Form1_Paint);
}
static void Main()
{
Application.Run(new Form1());
}
private void Form1_MouseUp(object sender, System.Windows.Forms.MouseEventArgs e)
{
Point mousePt = new Point(e.X, e.Y);
if(myPath.IsVisible(mousePt))
{
isImageClicked = true;
this.Text = "You clicked the strange shape...";
} else {
isImageClicked = false;
this.Text = "Images";
}
Invalidate();
}
private void Form1_Paint(object sender, System.Windows.Forms.PaintEventArgs e)
{
Graphics g = e.Graphics;
g.FillPath(Brushes.AliceBlue, myPath);
if(isImageClicked == true)
{
Pen outline = new Pen(Color.Black, 2);
g.DrawPath(outline, myPath);
}
}
}
Related examples in the same category