Mouse Buttons Click
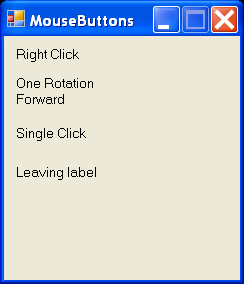
/*
Professional Windows GUI Programming Using C#
by Jay Glynn, Csaba Torok, Richard Conway, Wahid Choudhury,
Zach Greenvoss, Shripad Kulkarni, Neil Whitlow
Publisher: Peer Information
ISBN: 1861007663
*/
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace Wrox.WindowGUIProgramming.Chapter5
{
/// <summary>
/// Summary description for Form1.
/// </summary>
public class frmMouseButtons : System.Windows.Forms.Form
{
private System.Windows.Forms.Label lblLeftClick;
private System.Windows.Forms.Label lblRightClick;
private System.Windows.Forms.Label lblMiddleClick;
private System.Windows.Forms.Label lblHover;
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public frmMouseButtons()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
protected override void OnMouseDown(MouseEventArgs e)
{
switch(e.Button)
{
case(MouseButtons.Left):
lblLeftClick.Text = "Left Click";
break;
case(MouseButtons.Middle):
lblLeftClick.Text = "Middle Click";
break;
case(MouseButtons.Right):
lblLeftClick.Text = "Right Click";
break;
case(MouseButtons.XButton1):
lblLeftClick.Text = "XButton1 Click";
break;
case(MouseButtons.XButton2):
lblLeftClick.Text = "XButton2 Click";
break;
}
switch(e.Clicks)
{
case 1:
lblMiddleClick.Text = "Single Click";
break;
case 2:
lblMiddleClick.Text = "Double Click!";
break;
default:
lblMiddleClick.Text = "Many clicks!";
break;
}
}
protected override void OnMouseWheel(MouseEventArgs e)
{
switch(e.Delta)
{
case -360:
lblRightClick.Text = "One Rotation Reverse";
break;
case -720:
lblRightClick.Text = "Two Rotations Reverse";
break;
case 360:
lblRightClick.Text = "One Rotation Forward";
break;
case 720:
lblRightClick.Text = "Two Rotations Forward";
break;
default:
lblRightClick.Text = "Rotation wasn't full turn of wheel";
break;
}
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.lblLeftClick = new System.Windows.Forms.Label();
this.lblRightClick = new System.Windows.Forms.Label();
this.lblMiddleClick = new System.Windows.Forms.Label();
this.lblHover = new System.Windows.Forms.Label();
this.SuspendLayout();
//
// lblLeftClick
//
this.lblLeftClick.Location = new System.Drawing.Point(8, 8);
this.lblLeftClick.Name = "lblLeftClick";
this.lblLeftClick.TabIndex = 0;
//
// lblRightClick
//
this.lblRightClick.Location = new System.Drawing.Point(8, 32);
this.lblRightClick.Name = "lblRightClick";
this.lblRightClick.Size = new System.Drawing.Size(100, 32);
this.lblRightClick.TabIndex = 1;
//
// lblMiddleClick
//
this.lblMiddleClick.Location = new System.Drawing.Point(8, 72);
this.lblMiddleClick.Name = "lblMiddleClick";
this.lblMiddleClick.TabIndex = 2;
//
// lblHover
//
this.lblHover.Location = new System.Drawing.Point(8, 104);
this.lblHover.Name = "lblHover";
this.lblHover.TabIndex = 3;
this.lblHover.MouseEnter += new System.EventHandler(this.lblHover_MouseEnter);
this.lblHover.MouseHover += new System.EventHandler(this.lblHover_MouseHover);
this.lblHover.MouseLeave += new System.EventHandler(this.lblHover_MouseLeave);
//
// frmMouseButtons
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(184, 198);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.lblHover,
this.lblMiddleClick,
this.lblRightClick,
this.lblLeftClick});
this.MaximizeBox = false;
this.Name = "frmMouseButtons";
this.Text = "MouseButtons";
this.ResumeLayout(false);
}
#endregion
protected void lblHover_MouseEnter(object sender, EventArgs e)
{
lblRightClick.Text = "One Rotation Forward";
lblHover.Text = "Entering label";
Cursor = Cursors.NoMove2D;
}
protected void lblHover_MouseHover(object sender, EventArgs e)
{
lblHover.Text = "Hovering over label";
Cursor = Cursors.Hand;
System.Diagnostics.Debug.WriteLine("hover");
}
protected void lblHover_MouseLeave(object sender, EventArgs e)
{
lblHover.Text = "Leaving label";
Cursor = Cursors.Default;
}
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.Run(new frmMouseButtons());
}
}
}
Related examples in the same category