File.GetAccessControl Gets a FileSecurity object that encapsulates the access control list
using System;
using System.IO;
using System.Security.AccessControl;
class FileExample
{
public static void Main()
{
string fileName = "test.xml";
AddFileSecurity(fileName, @"DomainName\AccountName", FileSystemRights.ReadData, AccessControlType.Allow);
RemoveFileSecurity(fileName, @"DomainName\AccountName", FileSystemRights.ReadData, AccessControlType.Allow);
}
public static void AddFileSecurity(string fileName, string account, FileSystemRights rights, AccessControlType controlType)
{
FileSecurity fSecurity = File.GetAccessControl(fileName);
fSecurity.AddAccessRule(new FileSystemAccessRule(account, rights, controlType));
File.SetAccessControl(fileName, fSecurity);
}
public static void RemoveFileSecurity(string fileName, string account, FileSystemRights rights, AccessControlType controlType)
{
FileSecurity fSecurity = File.GetAccessControl(fileName);
fSecurity.RemoveAccessRule(new FileSystemAccessRule(account, rights, controlType));
File.SetAccessControl(fileName, fSecurity);
}
}
Related examples in the same category
1. | Get file Creation Time | | 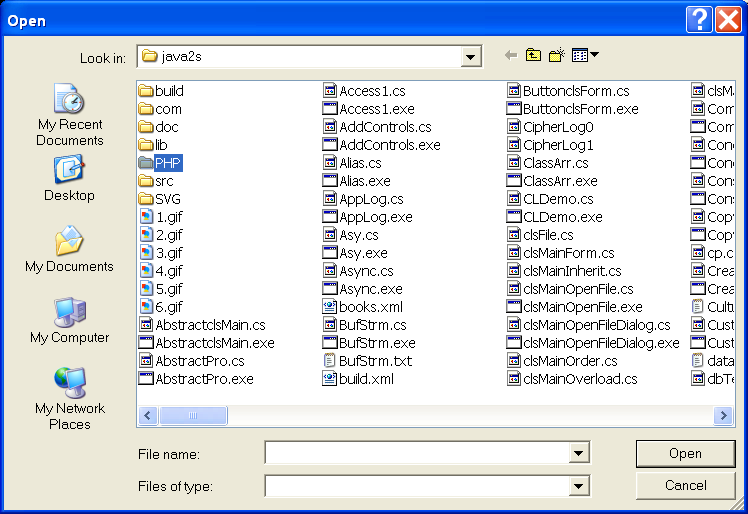 |
2. | Delete a file | | |
3. | Get File Access Control | | |
4. | illustrates the FileAttributes enumeration | | 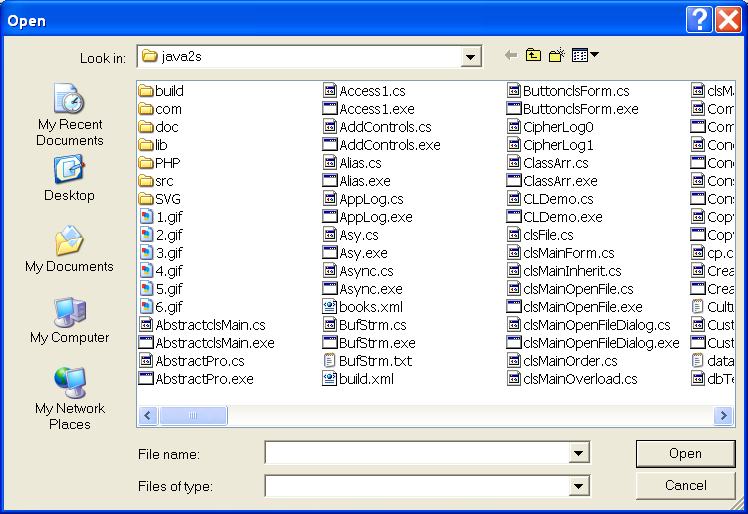 |
5. | illustrates the FileInfo class | | 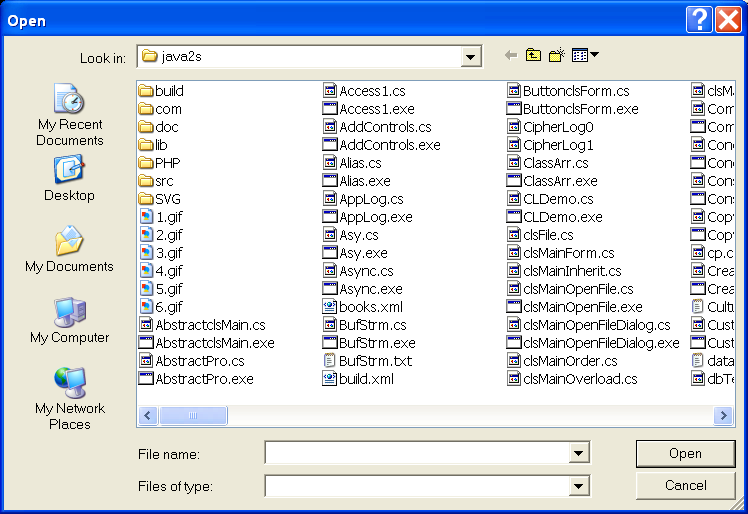 |
6. | illustrates the FileSystemWatcher class | | 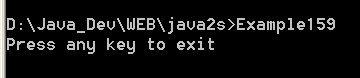 |
7. | File class to check whether a file exists, open and read | | |
8. | Uses methods in the File class to check whether a file exists | | |
9. | Uses methods in the File class to check the status of a file | | |
10. | File Class | | |
11. | File.AppendAllLines Method Appends lines to a file, and then closes the file. | | |
12. | File.AppendAllText Opens a file, appends the specified string to the file | | |
13. | File.AppendAllText Method Appends the string to the file, creating the file if it does not exist. | | |
14. | File.AppendText Creates a StreamWriter that appends UTF-8 encoded text to an existing file. | | |
15. | File.Copy Method Copies file to a new file | | |
16. | File.Create Creates or overwrites a file in the specified path. | | |
17. | File.Create Method (String, Int32) Creates or overwrites the specified file. | | |
18. | File.CreateText Method Creates or opens a file for writing UTF-8 encoded text. | | |
19. | File.Decrypt Decrypts a file | | |
20. | File.Exists Determines whether the specified file exists. | | |
21. | File.GetAttributes Method Gets the FileAttributes of the file on the path. | | |
22. | File.GetCreationTime Method Returns the creation date and time of the specified file or directory. | | |
23. | File.GetLastAccessTime Method Returns the date and time the specified file or directory was last accessed. | | |
24. | File.GetLastWriteTime Returns the date and time the specified file or directory was last written to. | | |
25. | File.Move Moves a file to a new location | | |
26. | File.Open Opens a FileStream on the specified path with read/write access. | | |
27. | File.Open Method (String, FileMode, FileAccess) | | |
28. | File.Open Method (String, FileMode, FileAccess, FileShare) | | |
29. | File.OpenRead Method Opens an existing file for reading. | | |
30. | File.OpenText Method Opens an existing UTF-8 encoded text file for reading. | | |
31. | File.OpenWrite Method Opens an existing file or creates a new file for writing. | | |
32. | File.ReadAllLines Method Opens a text file, reads all lines of the file, and then closes the file. | | |
33. | File.ReadAllLines Method (String, Encoding) | | |
34. | File.ReadLines Method | | |
35. | File.Replace Replaces contents of file | | |
36. | File.SetLastAccessTime Sets the date and time the specified file was last accessed. | | |
37. | File.SetLastWriteTime | | |