Bind key action to a form window
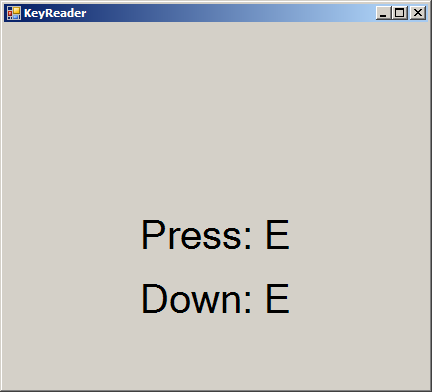
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
public class KeyReader : System.Windows.Forms.Form {
private System.Windows.Forms.Label lblPress;
private System.Windows.Forms.Label lblDown;
private System.Windows.Forms.Label label1;
public KeyReader() {
InitializeComponent();
}
private void InitializeComponent() {
this.lblPress = new System.Windows.Forms.Label();
this.lblDown = new System.Windows.Forms.Label();
this.label1 = new System.Windows.Forms.Label();
this.SuspendLayout();
this.lblPress.Font = new System.Drawing.Font("Microsoft Sans Serif", 30F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
this.lblPress.Location = new System.Drawing.Point(8, 190);
this.lblPress.Name = "lblPress";
this.lblPress.Size = new System.Drawing.Size(408, 48);
this.lblPress.TabIndex = 0;
this.lblPress.Text = "Press:";
this.lblPress.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
this.lblDown.Font = new System.Drawing.Font("Microsoft Sans Serif", 30F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
this.lblDown.Location = new System.Drawing.Point(8, 254);
this.lblDown.Name = "lblDown";
this.lblDown.Size = new System.Drawing.Size(408, 48);
this.lblDown.TabIndex = 0;
this.lblDown.Text = "Down:";
this.lblDown.TextAlign = System.Drawing.ContentAlignment.MiddleCenter;
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(424, 365);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.label1,
this.lblDown,
this.lblPress});
this.KeyPreview = true;
this.Name = "KeyReader";
this.Text = "KeyReader";
this.KeyDown += new System.Windows.Forms.KeyEventHandler(this.KeyReader_KeyDown);
this.KeyPress += new System.Windows.Forms.KeyPressEventHandler(this.KeyReader_KeyPress);
this.ResumeLayout(false);
}
[STAThread]
static void Main() {
Application.Run(new KeyReader());
}
private void KeyReader_KeyPress(object sender, System.Windows.Forms.KeyPressEventArgs e) {
lblPress.Text = "Press: " + Convert.ToString(e.KeyChar);
}
private void KeyReader_KeyDown(object sender, System.Windows.Forms.KeyEventArgs e) {
lblDown.Text = "Down: " + Convert.ToString(e.KeyCode);
if (e.KeyCode == Keys.ShiftKey){
MessageBox.Show("That is one shifty character");
}
}
}
Related examples in the same category