MDI Basics
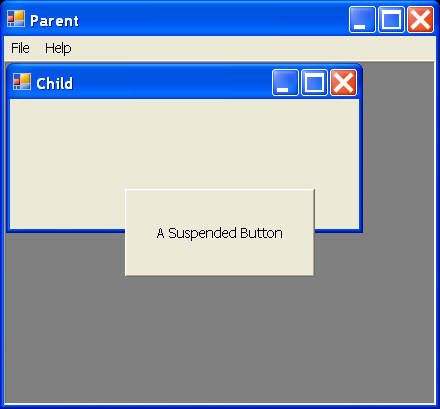
/*
User Interfaces in C#: Windows Forms and Custom Controls
by Matthew MacDonald
Publisher: Apress
ISBN: 1590590457
*/
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
namespace MDIBasics
{
/// <summary>
/// Summary description for Parent.
/// </summary>
public class Parent : System.Windows.Forms.Form
{
internal System.Windows.Forms.Button Button1;
internal System.Windows.Forms.MainMenu MainMenu1;
internal System.Windows.Forms.MenuItem MenuItem1;
internal System.Windows.Forms.MenuItem MenuItem2;
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public Parent()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if(components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.Button1 = new System.Windows.Forms.Button();
this.MainMenu1 = new System.Windows.Forms.MainMenu();
this.MenuItem1 = new System.Windows.Forms.MenuItem();
this.MenuItem2 = new System.Windows.Forms.MenuItem();
this.SuspendLayout();
//
// Button1
//
this.Button1.FlatStyle = System.Windows.Forms.FlatStyle.System;
this.Button1.Location = new System.Drawing.Point(82, 99);
this.Button1.Name = "Button1";
this.Button1.Size = new System.Drawing.Size(128, 68);
this.Button1.TabIndex = 2;
this.Button1.Text = "A Suspended Button";
//
// MainMenu1
//
this.MainMenu1.MenuItems.AddRange(new System.Windows.Forms.MenuItem[] {
this.MenuItem1,
this.MenuItem2});
//
// MenuItem1
//
this.MenuItem1.Index = 0;
this.MenuItem1.Text = "File";
//
// MenuItem2
//
this.MenuItem2.Index = 1;
this.MenuItem2.Text = "Help";
//
// Parent
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 14);
this.ClientSize = new System.Drawing.Size(292, 266);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.Button1});
this.Font = new System.Drawing.Font("Tahoma", 8.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
this.IsMdiContainer = true;
this.Menu = this.MainMenu1;
this.Name = "Parent";
this.Text = "Parent";
this.Load += new System.EventHandler(this.Parent_Load);
this.ResumeLayout(false);
}
#endregion
[STAThread]
static void Main()
{
Application.Run(new Parent());
}
private void Parent_Load(object sender, System.EventArgs e)
{
Child frmChild = new Child();
frmChild.MdiParent = this;
frmChild.Show();
}
}
/// <summary>
/// Summary description for Child.
/// </summary>
public class Child : System.Windows.Forms.Form
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public Child()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if(components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
//
// Child
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(236, 94);
this.Name = "Child";
this.Text = "Child";
}
#endregion
}
}
Related examples in the same category