Set MDI parent window
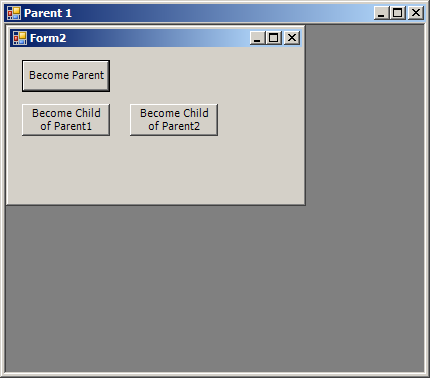
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
public class Form2 : Form
{
private System.Windows.Forms.Button Button3;
private System.Windows.Forms.Button Button2;
private System.Windows.Forms.Button Button1;
public Form2()
{
InitializeComponent();
}
private void InitializeComponent()
{
this.Button3 = new System.Windows.Forms.Button();
this.Button2 = new System.Windows.Forms.Button();
this.Button1 = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// Button3
//
this.Button3.Location = new System.Drawing.Point(120, 56);
this.Button3.Name = "Button3";
this.Button3.Size = new System.Drawing.Size(88, 32);
this.Button3.TabIndex = 8;
this.Button3.Text = "Become Child of Parent2";
this.Button3.Click += new System.EventHandler(this.Button3_Click);
//
// Button2
//
this.Button2.Location = new System.Drawing.Point(12, 56);
this.Button2.Name = "Button2";
this.Button2.Size = new System.Drawing.Size(88, 32);
this.Button2.TabIndex = 7;
this.Button2.Text = "Become Child of Parent1";
this.Button2.Click += new System.EventHandler(this.Button2_Click);
//
// Button1
//
this.Button1.Location = new System.Drawing.Point(12, 12);
this.Button1.Name = "Button1";
this.Button1.Size = new System.Drawing.Size(88, 32);
this.Button1.TabIndex = 6;
this.Button1.Text = "Become Parent";
this.Button1.Click += new System.EventHandler(this.Button1_Click);
//
// Form2
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(292, 154);
this.Controls.Add(this.Button3);
this.Controls.Add(this.Button2);
this.Controls.Add(this.Button1);
this.Font = new System.Drawing.Font("Tahoma", 8.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((byte)(0)));
this.Name = "Form2";
this.Text = "Form2";
this.ResumeLayout(false);
}
private void Button1_Click(object sender, System.EventArgs e)
{
this.Hide();
this.MdiParent = null;
this.IsMdiContainer = true;
this.Show();
}
private void Button2_Click(object sender, System.EventArgs e)
{
this.Hide();
this.IsMdiContainer = false;
this.MdiParent = Program.Main2;
this.Show();
}
private void Button3_Click(object sender, System.EventArgs e)
{
this.Hide();
this.IsMdiContainer = false;
this.MdiParent = Program.Main1;
this.Show();
}
}
public class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_FormClosed(object sender, FormClosedEventArgs e)
{
Application.Exit();
}
private void InitializeComponent()
{
this.SuspendLayout();
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(422, 351);
this.IsMdiContainer = true;
this.Name = "Form1";
this.Text = "Form1";
this.FormClosed += new System.Windows.Forms.FormClosedEventHandler(this.Form1_FormClosed);
this.ResumeLayout(false);
}
}
static class Program
{
public static Form1 Main1 = new Form1();
public static Form1 Main2 = new Form1();
public static Form2 Child = new Form2();
[STAThread]
public static void Main()
{
Application.EnableVisualStyles();
Main1.Text = "Parent 2";
Main2.Text = "Parent 1";
Main1.Show();
Main2.Show();
Child.MdiParent = Main2;
Child.Show();
System.Windows.Forms.Application.Run();
}
}
Related examples in the same category