Demonstrate passing an object to an event handler and performing the proper cast in the method
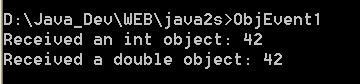
/*
C# Programming Tips & Techniques
by Charles Wright, Kris Jamsa
Publisher: Osborne/McGraw-Hill (December 28, 2001)
ISBN: 0072193794
*/
// ObjEvent.cs -- Demonstrate passing an object to an event handler and
// performing the proper cast in the method.
//
// Compile this program with the following command line:
// C:>csc ObjEvent.cs
using System;
namespace nsEvents
{
public class ObjEvent1
{
public delegate void EventHandler (object obj);
public event EventHandler EvInvoke;
public void FireEvent (object obj)
{
if (obj != null)
EvInvoke (obj);
}
static public void Main ()
{
ObjEvent1 main = new ObjEvent1 ();
main.EvInvoke = new ObjEvent1.EventHandler (ObjEvent);
main.FireEvent (42);
main.FireEvent (42.0);
}
static void ObjEvent (object obj)
{
if (obj is double)
{
Console.WriteLine ("Received a double object: " + (double) obj);
}
else if (obj is int)
{
Console.WriteLine ("Received an int object: " + (int) obj);
}
}
}
}
Related examples in the same category