illustrates the use of an event
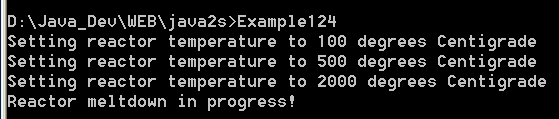
/*
Mastering Visual C# .NET
by Jason Price, Mike Gunderloy
Publisher: Sybex;
ISBN: 0782129110
*/
/*
Example12_4.cs illustrates the use of an event
*/
using System;
// declare the MeltdownEventArgs class (implements EventArgs)
class MeltdownEventArgs : EventArgs
{
// declare a private field named message
private string message;
// define a constructor
public MeltdownEventArgs(string message)
{
this.message = message;
}
// define a property to get the message
public string Message
{
get
{
return message;
}
}
}
// declare the Reactor class
class Reactor
{
// declare a private field named temperature
private int temperature;
// declare a delegate class named MeltdownHandler
public delegate void MeltdownHandler(
object reactor,
MeltdownEventArgs myMEA
);
// declare an event named OnMeltdown
public event MeltdownHandler OnMeltdown;
// define a property to set the temperature
public int Temperature
{
set
{
temperature = value;
// if the temperature is too high, the reactor melts down
if (temperature > 1000)
{
MeltdownEventArgs myMEA =
new MeltdownEventArgs("Reactor meltdown in progress!");
OnMeltdown(this, myMEA);
}
}
}
}
// declare the ReactorMonitor class
class ReactorMonitor
{
// define a constructor
public ReactorMonitor(Reactor myReactor)
{
myReactor.OnMeltdown +=
new Reactor.MeltdownHandler(DisplayMessage);
}
// define the DisplayMessage() method
public void DisplayMessage(
object myReactor, MeltdownEventArgs myMEA
)
{
Console.WriteLine(myMEA.Message);
}
}
public class Example12_4
{
public static void Main()
{
// create a Reactor object
Reactor myReactor = new Reactor();
// create a ReactorMonitor object
ReactorMonitor myReactorMonitor = new ReactorMonitor(myReactor);
// set myReactor.Temperature to 100 degrees Centigrade
Console.WriteLine("Setting reactor temperature to 100 degrees Centigrade");
myReactor.Temperature = 100;
// set myReactor.Temperature to 500 degrees Centigrade
Console.WriteLine("Setting reactor temperature to 500 degrees Centigrade");
myReactor.Temperature = 500;
// set myReactor.Temperature to 2000 degrees Centigrade
// (this causes the reactor to meltdown)
Console.WriteLine("Setting reactor temperature to 2000 degrees Centigrade");
myReactor.Temperature = 2000;
}
}
Related examples in the same category