How to create a custom attribute
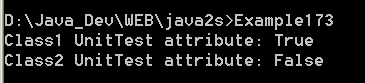
/*
Mastering Visual C# .NET
by Jason Price, Mike Gunderloy
Publisher: Sybex;
ISBN: 0782129110
*/
/*
Example17_3.cs shows how to create a custom attribute
*/
using System;
public class Example17_3
{
public static void Main()
{
UnitTest u;
// retrieve and display the UnitTest attributes of the classes
Console.Write("Class1 UnitTest attribute: ");
u = (UnitTest) Attribute.GetCustomAttribute(
typeof(Class1), typeof(UnitTest));
Console.WriteLine(u.Written());
Console.Write("Class2 UnitTest attribute: ");
u = (UnitTest) Attribute.GetCustomAttribute(
typeof(Class2), typeof(UnitTest));
Console.WriteLine(u.Written());
}
}
// declare an attribute named UnitTest
// UnitTest.Written is either true or false
public class UnitTest : Attribute
{
bool bWritten;
public bool Written()
{
return bWritten;
}
public UnitTest(bool Written)
{
bWritten = Written;
}
}
// apply the UnitTest attribute to two classes
[UnitTest(true)]
public class Class1
{
}
[UnitTest(false)]
public class Class2
{
}
Related examples in the same category