Use a named attribute parameter
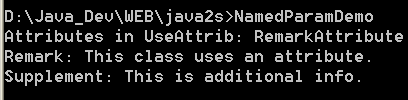
// Use a named attribute parameter.
using System;
using System.Reflection;
[AttributeUsage(AttributeTargets.All)]
class RemarkAttribute : Attribute {
string remarkValue; // underlies remark property
public string supplement; // this is a named parameter
public RemarkAttribute(string comment) {
remarkValue = comment;
supplement = "None";
}
public string remark {
get {
return remarkValue;
}
}
}
[RemarkAttribute("This class uses an attribute.",
supplement = "This is additional info.")]
class UseAttrib {
// ...
}
public class NamedParamDemo {
public static void Main() {
Type t = typeof(UseAttrib);
Console.Write("Attributes in " + t.Name + ": ");
object[] attribs = t.GetCustomAttributes(false);
foreach(object o in attribs) {
Console.WriteLine(o);
}
// Retrieve the RemarkAttribute.
Type tRemAtt = typeof(RemarkAttribute);
RemarkAttribute ra = (RemarkAttribute)
Attribute.GetCustomAttribute(t, tRemAtt);
Console.Write("Remark: ");
Console.WriteLine(ra.remark);
Console.Write("Supplement: ");
Console.WriteLine(ra.supplement);
}
}
Related examples in the same category